[GitHub] [servicecomb-service-center] tianxiaoliang commented on a change in pull request #789: Simple implementation of mongo cache for notify event to syncer
tianxiaoliang commented on a change in pull request #789: URL: https://github.com/apache/servicecomb-service-center/pull/789#discussion_r547747631 ## File path: datasource/mongo/sd/listwatch.go ## @@ -0,0 +1,45 @@ +/* + * Licensed to the Apache Software Foundation (ASF) under one or more + * contributor license agreements. See the NOTICE file distributed with + * this work for additional information regarding copyright ownership. + * The ASF licenses this file to You under the Apache License, Version 2.0 + * (the "License"); you may not use this file except in compliance with + * the License. You may obtain a copy of the License at + * + * http://www.apache.org/licenses/LICENSE-2.0 + * + * Unless required by applicable law or agreed to in writing, software + * distributed under the License is distributed on an "AS IS" BASIS, + * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. + * See the License for the specific language governing permissions and + * limitations under the License. + */ + +package sd + +import ( + "context" + "fmt" + "time" + + "go.mongodb.org/mongo-driver/bson" +) + +type ListWatchConfig struct { + Timeout time.Duration + Context context.Context +} + +func (lo *ListWatchConfig) String() string { + return fmt.Sprintf("{timeout: %s}", lo.Timeout) +} + +type ListWatch interface { + List(op ListWatchConfig) (*MongoListWatchResponse, error) + // not support new multiple watchers + Watch(op ListWatchConfig) Watcher + + DoWatch(ctx context.Context, f func(*MongoListWatchResponse)) error Review comment: 这些接口不能复用etcd么,不要绑定mongodb的概念,etcd和mongo应该有个共通的抽象。毕竟既然你已经用了mongodb了,还抽象什么内容呢 This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [servicecomb-service-center] tianxiaoliang commented on a change in pull request #789: Simple implementation of mongo cache for notify event to syncer
tianxiaoliang commented on a change in pull request #789: URL: https://github.com/apache/servicecomb-service-center/pull/789#discussion_r547747631 ## File path: datasource/mongo/sd/listwatch.go ## @@ -0,0 +1,45 @@ +/* + * Licensed to the Apache Software Foundation (ASF) under one or more + * contributor license agreements. See the NOTICE file distributed with + * this work for additional information regarding copyright ownership. + * The ASF licenses this file to You under the Apache License, Version 2.0 + * (the "License"); you may not use this file except in compliance with + * the License. You may obtain a copy of the License at + * + * http://www.apache.org/licenses/LICENSE-2.0 + * + * Unless required by applicable law or agreed to in writing, software + * distributed under the License is distributed on an "AS IS" BASIS, + * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. + * See the License for the specific language governing permissions and + * limitations under the License. + */ + +package sd + +import ( + "context" + "fmt" + "time" + + "go.mongodb.org/mongo-driver/bson" +) + +type ListWatchConfig struct { + Timeout time.Duration + Context context.Context +} + +func (lo *ListWatchConfig) String() string { + return fmt.Sprintf("{timeout: %s}", lo.Timeout) +} + +type ListWatch interface { + List(op ListWatchConfig) (*MongoListWatchResponse, error) + // not support new multiple watchers + Watch(op ListWatchConfig) Watcher + + DoWatch(ctx context.Context, f func(*MongoListWatchResponse)) error Review comment: 这些接口不能复用etcd么,不要绑定mongodb的概念,etcd和mongo应该有个共通的抽象 ## File path: datasource/mongo/sd/listwatch_inner.go ## @@ -0,0 +1,155 @@ +/* + * Licensed to the Apache Software Foundation (ASF) under one or more + * contributor license agreements. See the NOTICE file distributed with + * this work for additional information regarding copyright ownership. + * The ASF licenses this file to You under the Apache License, Version 2.0 + * (the "License"); you may not use this file except in compliance with + * the License. You may obtain a copy of the License at + * + * http://www.apache.org/licenses/LICENSE-2.0 + * + * Unless required by applicable law or agreed to in writing, software + * distributed under the License is distributed on an "AS IS" BASIS, + * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. + * See the License for the specific language governing permissions and + * limitations under the License. + */ + +package sd + +import ( + "context" + "fmt" + + "github.com/apache/servicecomb-service-center/datasource/mongo/client" + "github.com/apache/servicecomb-service-center/pkg/log" + "go.mongodb.org/mongo-driver/bson" + md "go.mongodb.org/mongo-driver/mongo" + "go.mongodb.org/mongo-driver/mongo/options" +) + +type innerListWatch struct { + Key string + resumeToken bson.Raw +} + +func (lw *innerListWatch) List(op ListWatchConfig) (*MongoListWatchResponse, error) { + otCtx, cancel := context.WithTimeout(op.Context, op.Timeout) + defer cancel() + + resp, err := client.GetMongoClient().Find(otCtx, lw.Key, bson.M{}) + if err != nil { + log.Error(fmt.Sprintf("list key %s failed", lw.Key), err) + return nil, err + } + + lwRsp := {} + lwRsp.Infos = make([]MongoInfo, 0) + for resp.Next(context.Background()) { + info := lw.doParseDocumentToMongoInfo(resp.Current) + lwRsp.Infos = append(lwRsp.Infos, info) + } + + return lwRsp, nil +} + +func (lw *innerListWatch) ResumeToken() bson.Raw { + return lw.resumeToken +} + +func (lw *innerListWatch) setResumeToken(resumeToken bson.Raw) { + lw.resumeToken = resumeToken +} + +func (lw *innerListWatch) Watch(op ListWatchConfig) Watcher { + return newInnerWatcher(lw, op) +} + +func (lw *innerListWatch) DoWatch(ctx context.Context, f func(*MongoListWatchResponse)) error { + csOptions := {} + csOptions.SetFullDocument(options.UpdateLookup) + + resumeToken := lw.ResumeToken() + if resumeToken != nil { + csOptions.SetResumeAfter(resumeToken) + } + + resp, err := client.GetMongoClient().Watch(ctx, lw.Key, md.Pipeline{}, csOptions) + + if err != nil { + log.Error(fmt.Sprintf("watch table %s failed", lw.Key), err) + f(nil) + return err + } + + for resp.Next(ctx) { + lwRsp := {} + + lw.setResumeToken(resp.ResumeToken()) + + wRsp := {} + err := bson.Unmarshal(resp.Current, ) + + if err != nil { + log.Error("error to parse bson raw to mongo watch response", err) + return err + } + +
[GitHub] [servicecomb-service-center] fuziye01 commented on a change in pull request #789: Simple implementation of mongo cache for notify event to syncer
fuziye01 commented on a change in pull request #789: URL: https://github.com/apache/servicecomb-service-center/pull/789#discussion_r547744903 ## File path: datasource/mongo/sd/cache.go ## @@ -0,0 +1,46 @@ +/* +* Licensed to the Apache Software Foundation (ASF) under one or more +* contributor license agreements. See the NOTICE file distributed with +* this work for additional information regarding copyright ownership. +* The ASF licenses this file to You under the Apache License, Version 2.0 +* (the "License"); you may not use this file except in compliance with +* the License. You may obtain a copy of the License at +* +* http://www.apache.org/licenses/LICENSE-2.0 +* +* Unless required by applicable law or agreed to in writing, software +* distributed under the License is distributed on an "AS IS" BASIS, +* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. +* See the License for the specific language governing permissions and +* limitations under the License. + */ + +package sd + +// Cache stores db data. +type Cache interface { + CacheReader + + Put(id string, v interface{}) + + Remove(id string) + + MarkDirty() + + Dirty() bool + + Clear() Review comment: Dirty()用来判断缓存是否需要reset,reset的步骤包含了 1. 清空缓存,即Clear() 2.重建缓存 This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [servicecomb-java-chassis] liubao68 commented on issue #2158: Consumer调用Producer超时,会暴露内部服务器地址
liubao68 commented on issue #2158: URL: https://github.com/apache/servicecomb-java-chassis/issues/2158#issuecomment-749982195 不存在, 加一个就可以了。 This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [servicecomb-service-center] fuziye01 commented on a change in pull request #789: Simple implementation of mongo cache for notify event to syncer
fuziye01 commented on a change in pull request #789: URL: https://github.com/apache/servicecomb-service-center/pull/789#discussion_r547736199 ## File path: datasource/mongo/mongo.go ## @@ -118,3 +121,12 @@ func (ds *DataSource) createIndexes() (err error) { } return } + +func (ds *DataSource) initStore() { + if !config.GetRegistry().EnableCache { + log.Debug("cache is disabled") + return + } + sd.Store().Run() + <-sd.Store().Ready() Review comment: 此处是等待缓存加载完 This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [servicecomb-service-center] tianxiaoliang commented on a change in pull request #789: Simple implementation of mongo cache for notify event to syncer
tianxiaoliang commented on a change in pull request #789: URL: https://github.com/apache/servicecomb-service-center/pull/789#discussion_r547732860 ## File path: datasource/mongo/sd/event_proxy.go ## @@ -0,0 +1,69 @@ +/* + * Licensed to the Apache Software Foundation (ASF) under one or more + * contributor license agreements. See the NOTICE file distributed with + * this work for additional information regarding copyright ownership. + * The ASF licenses this file to You under the Apache License, Version 2.0 + * (the "License"); you may not use this file except in compliance with + * the License. You may obtain a copy of the License at + * + * http://www.apache.org/licenses/LICENSE-2.0 + * + * Unless required by applicable law or agreed to in writing, software + * distributed under the License is distributed on an "AS IS" BASIS, + * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. + * See the License for the specific language governing permissions and + * limitations under the License. + */ + +package sd + +import ( + "fmt" + "sync" + + "github.com/apache/servicecomb-service-center/pkg/log" + "github.com/apache/servicecomb-service-center/pkg/util" +) + +var ( + eventProxies = make(map[string]*MongoEventProxy) +) + +type MongoEventProxy struct { + evtHandleFuncs []MongoEventFunc + lock sync.RWMutex +} + +func (h *MongoEventProxy) AddHandleFunc(f MongoEventFunc) { + h.lock.Lock() + h.evtHandleFuncs = append(h.evtHandleFuncs, f) + h.lock.Unlock() +} + +func (h *MongoEventProxy) OnEvent(evt MongoEvent) { + h.lock.RLock() + for _, f := range h.evtHandleFuncs { + f(evt) + } + h.lock.RUnlock() +} + +// InjectConfig will inject a resource changed event callback function in Config +func (h *MongoEventProxy) InjectConfig(cfg *Config) *Config { Review comment: 这个方法只需要传入回调函数,不要传入结构体,变量意义不明,方法意义不明,注释确实很明确,但是提倡方法本身就是明确的 This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [servicecomb-service-center] xiangyanggong opened a new issue #790: sc连接etcd安全认证
xiangyanggong opened a new issue #790: URL: https://github.com/apache/servicecomb-service-center/issues/790 如果sc后端使用独立的etcd集群,而该集群开启了安全认证功能,那sc该如何配置呢?在sc的配置文件中没有看到etcd用户密码的配置项 This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [servicecomb-java-chassis] liubao68 commented on issue #2160: CSE压测REST接口出现大量590错误码
liubao68 commented on issue #2160: URL: https://github.com/apache/servicecomb-java-chassis/issues/2160#issuecomment-749975970 590是服务端错误的意思, 一般都会打印错误日志,可以检查下日志是否有异常。 590错误和并发连接数没有必然联系,需要具体问题具体分析。 [异常处理](https://docs.servicecomb.io/java-chassis/zh_CN/general-development/error-handling/) 有介绍什么时候抛出 590错误。 This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [servicecomb-service-center] tianxiaoliang commented on a change in pull request #789: Simple implementation of mongo cache for notify event to syncer
tianxiaoliang commented on a change in pull request #789: URL: https://github.com/apache/servicecomb-service-center/pull/789#discussion_r547730463 ## File path: datasource/mongo/sd/watcher.go ## @@ -0,0 +1,23 @@ +/* + * Licensed to the Apache Software Foundation (ASF) under one or more + * contributor license agreements. See the NOTICE file distributed with + * this work for additional information regarding copyright ownership. + * The ASF licenses this file to You under the Apache License, Version 2.0 + * (the "License"); you may not use this file except in compliance with + * the License. You may obtain a copy of the License at + * + * http://www.apache.org/licenses/LICENSE-2.0 + * + * Unless required by applicable law or agreed to in writing, software + * distributed under the License is distributed on an "AS IS" BASIS, + * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. + * See the License for the specific language governing permissions and + * limitations under the License. + */ + +package sd + +type Watcher interface { + EventBus() <-chan *MongoListWatchResponse Review comment: 这个方法名肯定没有达意,eventbus是消息总线 ## File path: datasource/mongo/sd/event_proxy.go ## @@ -0,0 +1,69 @@ +/* + * Licensed to the Apache Software Foundation (ASF) under one or more + * contributor license agreements. See the NOTICE file distributed with + * this work for additional information regarding copyright ownership. + * The ASF licenses this file to You under the Apache License, Version 2.0 + * (the "License"); you may not use this file except in compliance with + * the License. You may obtain a copy of the License at + * + * http://www.apache.org/licenses/LICENSE-2.0 + * + * Unless required by applicable law or agreed to in writing, software + * distributed under the License is distributed on an "AS IS" BASIS, + * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. + * See the License for the specific language governing permissions and + * limitations under the License. + */ + +package sd + +import ( + "fmt" + "sync" + + "github.com/apache/servicecomb-service-center/pkg/log" + "github.com/apache/servicecomb-service-center/pkg/util" +) + +var ( + eventProxies = make(map[string]*MongoEventProxy) +) + +type MongoEventProxy struct { + evtHandleFuncs []MongoEventFunc Review comment: 没特别理由用sync.Map ## File path: datasource/mongo/sd/watcher_inner.go ## @@ -0,0 +1,87 @@ +/* + * Licensed to the Apache Software Foundation (ASF) under one or more + * contributor license agreements. See the NOTICE file distributed with + * this work for additional information regarding copyright ownership. + * The ASF licenses this file to You under the Apache License, Version 2.0 + * (the "License"); you may not use this file except in compliance with + * the License. You may obtain a copy of the License at + * + * http://www.apache.org/licenses/LICENSE-2.0 + * + * Unless required by applicable law or agreed to in writing, software + * distributed under the License is distributed on an "AS IS" BASIS, + * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. + * See the License for the specific language governing permissions and + * limitations under the License. + */ + +package sd + +import ( + "context" + "sync" + + "github.com/apache/servicecomb-service-center/pkg/gopool" + "github.com/apache/servicecomb-service-center/pkg/log" +) + +type innerWatcher struct { + CfgListWatchConfig + buschan *MongoListWatchResponse Review comment: MongoListWatchResponse 并不是一个总线的名字,如果你期望要一个总线,就抽象一个struct,别拿变量表示总线 This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [servicecomb-service-center] tianxiaoliang commented on a change in pull request #789: Simple implementation of mongo cache for notify event to syncer
tianxiaoliang commented on a change in pull request #789: URL: https://github.com/apache/servicecomb-service-center/pull/789#discussion_r547724805 ## File path: datasource/mongo/sd/common.go ## @@ -0,0 +1,36 @@ +/* +* Licensed to the Apache Software Foundation (ASF) under one or more +* contributor license agreements. See the NOTICE file distributed with +* this work for additional information regarding copyright ownership. +* The ASF licenses this file to You under the Apache License, Version 2.0 +* (the "License"); you may not use this file except in compliance with +* the License. You may obtain a copy of the License at +* +* http://www.apache.org/licenses/LICENSE-2.0 +* +* Unless required by applicable law or agreed to in writing, software +* distributed under the License is distributed on an "AS IS" BASIS, +* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. +* See the License for the specific language governing permissions and +* limitations under the License. + */ + +package sd + +import ( + "time" +) + +const ( + // force re-list + DefaultForceListInterval = 10 + + minWaitInterval = 1 * time.Second + eventBlockSize = 1000 + eventBusSize= 1000 + + INSERT = "insert" + UPDATE = "update" Review comment: 驼峰才是go风格的常量命 ## File path: datasource/mongo/sd/config.go ## @@ -0,0 +1,73 @@ +/* + * Licensed to the Apache Software Foundation (ASF) under one or more + * contributor license agreements. See the NOTICE file distributed with + * this work for additional information regarding copyright ownership. + * The ASF licenses this file to You under the Apache License, Version 2.0 + * (the "License"); you may not use this file except in compliance with + * the License. You may obtain a copy of the License at + * + * http://www.apache.org/licenses/LICENSE-2.0 + * + * Unless required by applicable law or agreed to in writing, software + * distributed under the License is distributed on an "AS IS" BASIS, + * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. + * See the License for the specific language governing permissions and + * limitations under the License. + */ + +package sd + +import ( + "fmt" + "time" +) + +const ( + DefaultTimeout = 30 * time.Second + DefaultCacheInitSize = 100 +) + +type Config struct { Review comment: 统一风格叫Options ## File path: datasource/mongo/sd/config.go ## @@ -0,0 +1,73 @@ +/* + * Licensed to the Apache Software Foundation (ASF) under one or more + * contributor license agreements. See the NOTICE file distributed with + * this work for additional information regarding copyright ownership. + * The ASF licenses this file to You under the Apache License, Version 2.0 + * (the "License"); you may not use this file except in compliance with + * the License. You may obtain a copy of the License at + * + * http://www.apache.org/licenses/LICENSE-2.0 + * + * Unless required by applicable law or agreed to in writing, software + * distributed under the License is distributed on an "AS IS" BASIS, + * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. + * See the License for the specific language governing permissions and + * limitations under the License. + */ + +package sd + +import ( + "fmt" + "time" +) + +const ( + DefaultTimeout = 30 * time.Second + DefaultCacheInitSize = 100 +) + +type Config struct { + // Key is the table to unique specify resource type + Key string + InitSize int + Timeout time.Duration + Period time.Duration + OnEvent MongoEventFunc +} + +func (cfg *Config) String() string { + return fmt.Sprintf("{key: %s, timeout: %s, period: %s}", + cfg.Key, cfg.Timeout, cfg.Period) +} + +func (cfg *Config) WithTable(key string) *Config { + cfg.Key = key + return cfg +} + +func (cfg *Config) WithEventFunc(f MongoEventFunc) *Config { + cfg.OnEvent = f + return cfg +} + +func (cfg *Config) AppendEventFunc(f MongoEventFunc) *Config { + if prev := cfg.OnEvent; prev != nil { + next := f + f = func(evt MongoEvent) { + prev(evt) + next(evt) + } + } + cfg.OnEvent = f + return cfg +} + +func Configure() *Config { Review comment: 这方法名叫DefaultOptions ## File path: datasource/mongo/sd/config.go ## @@ -0,0 +1,73 @@ +/* + * Licensed to the Apache Software Foundation (ASF) under one or more + * contributor license agreements. See the NOTICE file distributed with + * this work for additional information regarding copyright ownership. + * The ASF licenses this file to You under the Apache License, Version 2.0 + * (the "License"); you may not use this file except in compliance with + * the License. You may obtain a
[GitHub] [servicecomb-service-center] tianxiaoliang commented on a change in pull request #789: Simple implementation of mongo cache for notify event to syncer
tianxiaoliang commented on a change in pull request #789: URL: https://github.com/apache/servicecomb-service-center/pull/789#discussion_r547683695 ## File path: datasource/mongo/mongo.go ## @@ -118,3 +121,12 @@ func (ds *DataSource) createIndexes() (err error) { } return } + +func (ds *DataSource) initStore() { + if !config.GetRegistry().EnableCache { + log.Debug("cache is disabled") + return + } + sd.Store().Run() + <-sd.Store().Ready() Review comment: 为何要等着信号到来 ## File path: datasource/mongo/sd/cache.go ## @@ -0,0 +1,46 @@ +/* +* Licensed to the Apache Software Foundation (ASF) under one or more +* contributor license agreements. See the NOTICE file distributed with +* this work for additional information regarding copyright ownership. +* The ASF licenses this file to You under the Apache License, Version 2.0 +* (the "License"); you may not use this file except in compliance with +* the License. You may obtain a copy of the License at +* +* http://www.apache.org/licenses/LICENSE-2.0 +* +* Unless required by applicable law or agreed to in writing, software +* distributed under the License is distributed on an "AS IS" BASIS, +* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. +* See the License for the specific language governing permissions and +* limitations under the License. + */ + +package sd + +// Cache stores db data. +type Cache interface { + CacheReader + + Put(id string, v interface{}) + + Remove(id string) + + MarkDirty() + + Dirty() bool + + Clear() Review comment: 为何将两个接口分离 ## File path: datasource/mongo/sd/cache.go ## @@ -0,0 +1,46 @@ +/* +* Licensed to the Apache Software Foundation (ASF) under one or more +* contributor license agreements. See the NOTICE file distributed with +* this work for additional information regarding copyright ownership. +* The ASF licenses this file to You under the Apache License, Version 2.0 +* (the "License"); you may not use this file except in compliance with +* the License. You may obtain a copy of the License at +* +* http://www.apache.org/licenses/LICENSE-2.0 +* +* Unless required by applicable law or agreed to in writing, software +* distributed under the License is distributed on an "AS IS" BASIS, +* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. +* See the License for the specific language governing permissions and +* limitations under the License. + */ + +package sd + +// Cache stores db data. +type Cache interface { + CacheReader + + Put(id string, v interface{}) + + Remove(id string) + + MarkDirty() + + Dirty() bool + + Clear() Review comment: 不可能一个缓存写,另个一缓存读 This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [servicecomb-java-chassis] NoComments commented on issue #2158: Consumer调用Producer超时,会暴露内部服务器地址
NoComments commented on issue #2158: URL: https://github.com/apache/servicecomb-java-chassis/issues/2158#issuecomment-749964497 2.1.0~2.1.3中不存在TimeoutExceptionConverter这个类 ![Uploading image.png…]() This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [servicecomb-java-chassis] myVictor closed issue #2139: @RequestBody 启动报错2.x
myVictor closed issue #2139: URL: https://github.com/apache/servicecomb-java-chassis/issues/2139 This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [servicecomb-java-chassis] huangdong-new opened a new issue #2160: CSE压测REST接口出现大量590错误码
huangdong-new opened a new issue #2160: URL: https://github.com/apache/servicecomb-java-chassis/issues/2160 背景介绍: 使用Jmeter工具压测REST接口性能,Jmeter工具设置了1600个线程并发,REST请求是通过SLB转发给后端的服务。后端服务通信协议是Vertx。 问题描述: 启动压测时,后端服务返回大量的590错误,REST请求并未走到后端服务的业务处理逻辑中。几分钟后590错误还是会偶尔出现。 搜索业务运行日志并未发现任何ERROR级别日志打印,但在接口日志中可以看到590的错误返回码。 在网上搜索说请求数超过并发连接数会返回590的错误码,但是我在CSE开发指导文档中没有搜索到设置并发连接数的地方。 This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [servicecomb-service-center] fuziye01 commented on a change in pull request #789: Simple implementation of mongo cache for notify event to syncer
fuziye01 commented on a change in pull request #789: URL: https://github.com/apache/servicecomb-service-center/pull/789#discussion_r547591974 ## File path: datasource/mongo/mongo.go ## @@ -23,6 +23,7 @@ import ( "github.com/apache/servicecomb-service-center/datasource" "github.com/apache/servicecomb-service-center/datasource/mongo/client" "github.com/apache/servicecomb-service-center/datasource/mongo/heartbeat" + "github.com/apache/servicecomb-service-center/datasource/mongo/sd" Review comment: service discovery This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [servicecomb-java-chassis] tangfan916 commented on issue #2156: serviceComb版本是1.3.0,vertx-core版本从3.6.3升级到3.9.4 微服务间调用异常 NoSuchMethodError: io.vertx.core.http.RequestOptions.setSsl(Z)Lio/vertx
tangfan916 commented on issue #2156: URL: https://github.com/apache/servicecomb-java-chassis/issues/2156#issuecomment-749864832 io.vertx.core.http.RequestOptions.setSsl() 新版本是存在的,变化只是把参数类型从boolean变为Boolean This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [servicecomb-service-center] jasononion commented on a change in pull request #789: Simple implementation of mongo cache for notify event to syncer
jasononion commented on a change in pull request #789: URL: https://github.com/apache/servicecomb-service-center/pull/789#discussion_r547176271 ## File path: datasource/mongo/mongo.go ## @@ -118,3 +121,12 @@ func (ds *DataSource) createIndexes() (err error) { } return } + +func (ds *DataSource) initStore() { + if !config.GetRegistry().EnableCache { + log.Debug("cache enable is false") Review comment: cache is disabled ## File path: datasource/mongo/sd/typestore_test.go ## @@ -0,0 +1,43 @@ +package sd + +import ( + "testing" + + "github.com/stretchr/testify/assert" +) + +func TestTypeStore_Initialize(t *testing.T) { + s := TypeStore{} + s.Initialize() + assert.NotNil(t, s.ready) + assert.NotNil(t, s.goroutine) + assert.Equal(t, false, s.isClose) +} + +func TestTypeStore_Ready(t *testing.T) { + s := TypeStore{} + s.Initialize() + c := s.Ready() + assert.NotNil(t, c) + //s.Run() Review comment: 注释代码去掉 ## File path: datasource/mongo/mongo.go ## @@ -23,6 +23,7 @@ import ( "github.com/apache/servicecomb-service-center/datasource" "github.com/apache/servicecomb-service-center/datasource/mongo/client" "github.com/apache/servicecomb-service-center/datasource/mongo/heartbeat" + "github.com/apache/servicecomb-service-center/datasource/mongo/sd" Review comment: sd 的包名含义是? This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [servicecomb-java-chassis] liubao68 commented on issue #2159: Apache Commons Configuration-1.10 满分致命漏洞
liubao68 commented on issue #2159: URL: https://github.com/apache/servicecomb-java-chassis/issues/2159#issuecomment-749550759 servicecomb using 1.10, not 2.0.2 This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [servicecomb-java-chassis] liubao68 edited a comment on issue #2158: Consumer调用Producer超时,会暴露内部服务器地址
liubao68 edited a comment on issue #2158: URL: https://github.com/apache/servicecomb-java-chassis/issues/2158#issuecomment-749507191 这个异常是 [TimeoutExceptionConverter](https://github.com/apache/servicecomb-java-chassis/blob/master/core/src/main/java/org/apache/servicecomb/core/exception/converter/TimeoutExceptionConverter.java) 返回的, 你可以定制和覆盖默认实现。 服务之间调用的消息已经去掉了各种用户输入,不会有信息泄露问题。 但是想这种更加严格的信息泄露要求, 建议产品可以在外部服务(比如网关)做过滤器,防止异常情况下,携带任何内部信息, 不过这样也可能给问题定位带来很大的麻烦。 This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [servicecomb-java-chassis] Neverstop commented on issue #2159: Apache Commons Configuration-1.10 满分致命漏洞
Neverstop commented on issue #2159: URL: https://github.com/apache/servicecomb-java-chassis/issues/2159#issuecomment-749527859 好的 了解了 谢谢 This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [servicecomb-java-chassis] Neverstop closed issue #2159: Apache Commons Configuration-1.10 满分致命漏洞
Neverstop closed issue #2159: URL: https://github.com/apache/servicecomb-java-chassis/issues/2159 This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [servicecomb-java-chassis] jeho0815 commented on issue #2159: Apache Commons Configuration-1.10 满分致命漏洞
jeho0815 commented on issue #2159: URL: https://github.com/apache/servicecomb-java-chassis/issues/2159#issuecomment-749526814 Apache Commons Configuration可以使用yaml配置,它是利用第三方来解析,servicecomb本身解析yaml是直接使用snakeyaml的,还有解析的yaml来源是否可信,如果你的来源都是可信的,也可以认为不涉及 This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [servicecomb-java-chassis] lengshanwudi commented on issue #2151: 接口日志在URI尾部拼接特殊字符,该特殊字符产生传递拼接问题
lengshanwudi commented on issue #2151: URL: https://github.com/apache/servicecomb-java-chassis/issues/2151#issuecomment-749523512 多谢:) This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [servicecomb-java-chassis] lengshanwudi closed issue #2151: 接口日志在URI尾部拼接特殊字符,该特殊字符产生传递拼接问题
lengshanwudi closed issue #2151: URL: https://github.com/apache/servicecomb-java-chassis/issues/2151 This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [servicecomb-java-chassis] Neverstop commented on issue #2159: Apache Commons Configuration-1.10 满分致命漏洞
Neverstop commented on issue #2159: URL: https://github.com/apache/servicecomb-java-chassis/issues/2159#issuecomment-749521669 uses a third-party library to parse YAML 咨询一下这个组件到底是什么 This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [servicecomb-java-chassis] jeho0815 commented on issue #2159: Apache Commons Configuration-1.10 满分致命漏洞
jeho0815 commented on issue #2159: URL: https://github.com/apache/servicecomb-java-chassis/issues/2159#issuecomment-749519025 Apache Commons Configuration uses a third-party library to parse YAML files which by default allows the instantiation of classes if the YAML 没用到这个解析yaml,使用的是snakeyaml,所以不涉及 This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [servicecomb-service-center] lilai23 commented on a change in pull request #789: Simple implementation of mongo cache for notify event to syncer
lilai23 commented on a change in pull request #789: URL: https://github.com/apache/servicecomb-service-center/pull/789#discussion_r547250190 ## File path: datasource/mongo/sd/cache.go ## @@ -0,0 +1,46 @@ +///* +// * Licensed to the Apache Software Foundation (ASF) under one or more +// * contributor license agreements. See the NOTICE file distributed with +// * this work for additional information regarding copyright ownership. +// * The ASF licenses this file to You under the Apache License, Version 2.0 Review comment: 注释多了// ## File path: datasource/mongo/sd/common.go ## @@ -0,0 +1,34 @@ +// Licensed to the Apache Software Foundation (ASF) under one or more +// contributor license agreements. See the NOTICE file distributed with +// this work for additional information regarding copyright ownership. +// The ASF licenses this file to You under the Apache License, Version 2.0 +// (the "License"); you may not use this file except in compliance with +// the License. You may obtain a copy of the License at +// +// http://www.apache.org/licenses/LICENSE-2.0 Review comment: 这边注释应该用/* */吧 This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [servicecomb-java-chassis] Neverstop opened a new issue #2159: Apache Commons Configuration-1.10 满分致命漏洞
Neverstop opened a new issue #2159: URL: https://github.com/apache/servicecomb-java-chassis/issues/2159 https://nvd.nist.gov/vuln/detail/CVE-2020-1953#vulnCurrentDescriptionTitle servicecomb使用版本:2.0.2 请问这个漏洞涉及吗 This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [servicecomb-java-chassis] liubao68 commented on a change in pull request #2154: Edge's 490 exception is thrown and can be used for business processing
liubao68 commented on a change in pull request #2154: URL: https://github.com/apache/servicecomb-java-chassis/pull/2154#discussion_r547244883 ## File path: transports/transport-rest/transport-rest-client/src/main/java/org/apache/servicecomb/transport/rest/client/http/RestClientInvocation.java ## @@ -116,12 +118,17 @@ public void invoke(Invocation invocation, AsyncResponse asyncResp) throws Except filter.beforeSendRequest(invocation, requestEx); } } +List httpRestClientInvocationHooks = +SPIServiceUtils.getAllService(HttpRestClientInvocationHook.class); clientRequest.exceptionHandler(e -> { invocation.getTraceIdLogger() .error(LOGGER, "Failed to send request, alreadyFailed:{}, local:{}, remote:{}, message={}.", alreadyFailed, getLocalAddress(), ipPort.getSocketAddress(), ExceptionUtils.getExceptionMessageWithoutTrace(e)); + httpRestClientInvocationHooks.forEach(httpServerExceptionHandler -> { Review comment: ``` public class MyHandler implements Handler { private static final Logger LOGGER = LoggerFactory.getLogger(MyHandler.class); @Override public void handle(Invocation invocation, AsyncResponse asyncResp) throws Exception { LOGGER.info("before"); invocation.next(response -> { LOGGER.info("after"); }); } } ``` You can find many Handler implementations in servicecomb-java-chassis project. This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [servicecomb-java-chassis] liubao68 commented on a change in pull request #2154: Edge's 490 exception is thrown and can be used for business processing
liubao68 commented on a change in pull request #2154: URL: https://github.com/apache/servicecomb-java-chassis/pull/2154#discussion_r547244883 ## File path: transports/transport-rest/transport-rest-client/src/main/java/org/apache/servicecomb/transport/rest/client/http/RestClientInvocation.java ## @@ -116,12 +118,17 @@ public void invoke(Invocation invocation, AsyncResponse asyncResp) throws Except filter.beforeSendRequest(invocation, requestEx); } } +List httpRestClientInvocationHooks = +SPIServiceUtils.getAllService(HttpRestClientInvocationHook.class); clientRequest.exceptionHandler(e -> { invocation.getTraceIdLogger() .error(LOGGER, "Failed to send request, alreadyFailed:{}, local:{}, remote:{}, message={}.", alreadyFailed, getLocalAddress(), ipPort.getSocketAddress(), ExceptionUtils.getExceptionMessageWithoutTrace(e)); + httpRestClientInvocationHooks.forEach(httpServerExceptionHandler -> { Review comment: ``` public class MyHandler implements Handler { private static final Logger LOGGER = LoggerFactory.getLogger(MyHandler.class); public static final String SPLITPARAM_RESPONSE_USER_SUFFIX = "(modified by MyHandler)"; @Override public void handle(Invocation invocation, AsyncResponse asyncResp) throws Exception { LOGGER.info("before"); invocation.next(response -> { LOGGER.info("after"); }); } } ``` You can find many Handler implementations in servicecomb-java-chassis project. This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [servicecomb-java-chassis] liubao68 edited a comment on issue #2158: Consumer调用Producer超时,会暴露内部服务器地址
liubao68 edited a comment on issue #2158: URL: https://github.com/apache/servicecomb-java-chassis/issues/2158#issuecomment-749507191 这个异常是 TimeoutExceptioinConverter 返回的, 你可以定制和覆盖默认实现。 服务之间调用的消息已经去掉了各种用户输入,不会有信息泄露问题。 但是想这种更加严格的信息泄露要求, 建议产品可以在外部服务(比如网关)做过滤器,防止异常情况下,携带任何内部信息, 不过这样也可能给问题定位带来很大的麻烦。 This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [servicecomb-java-chassis] liubao68 commented on issue #2158: Consumer调用Producer超时,会暴露内部服务器地址
liubao68 commented on issue #2158: URL: https://github.com/apache/servicecomb-java-chassis/issues/2158#issuecomment-749507191 这个异常是 TimeoutExceptioinConverter 返回的, 你可以定制和覆盖默认实现。 This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [servicecomb-java-chassis] NoComments opened a new issue #2158: Consumer调用Producer超时,会暴露内部服务器地址
NoComments opened a new issue #2158: URL: https://github.com/apache/servicecomb-java-chassis/issues/2158 Consumer在调用Producer服务超时会返回以下信息给前端,在消息中会暴露内网服务器地址,如下图: 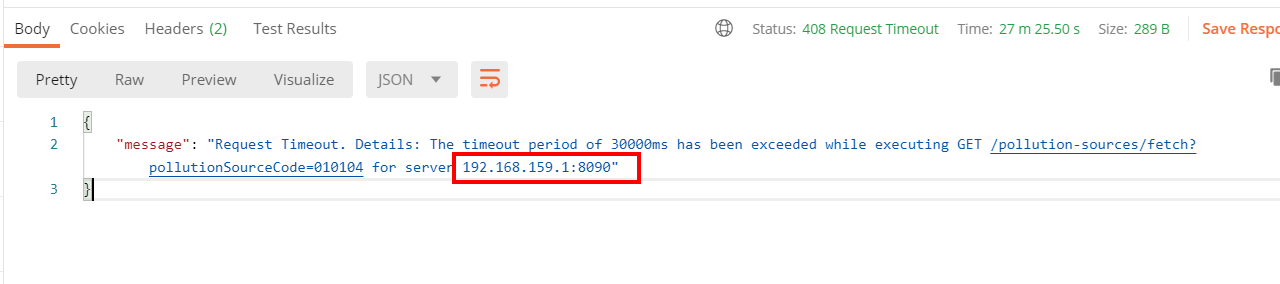 经排查,定位到是RestClientInvocation中对Vertx的超时异常NoStackTraceTimeoutException没有做特殊处理,直接把Vertx的异常信息返回给前端。 请问这种问题如何解决? This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [servicecomb-java-chassis] liubao68 edited a comment on issue #1587: @RestControllerAdvice配置失效问题
liubao68 edited a comment on issue #1587: URL: https://github.com/apache/servicecomb-java-chassis/issues/1587#issuecomment-749445912 是否能捕获到,要看异常抛出的位置。 这个异常ExceptionToProducerResponseConverter是捕获不到的。 目前java-chassis不能很好的由业务处理这类异常。 java-chassis 的处理流程会进行一些重构,来解决这类边角问题,不过是一个大版本规划,短期内不会解决。 你可以针对这个场景提一个issue。 This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [servicecomb-java-chassis] liubao68 commented on issue #1587: @RestControllerAdvice配置失效问题
liubao68 commented on issue #1587: URL: https://github.com/apache/servicecomb-java-chassis/issues/1587#issuecomment-749445912 是否能捕获到,要看异常抛出的位置。 这个异常ExceptionToProducerResponseConverter是捕获不到的。 目前java-chassis不能很好的由业务处理这类异常。 This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[GitHub] [servicecomb-java-chassis] wujimin merged pull request #2157: [SCB-2169] optimize filter declare and chain config
wujimin merged pull request #2157: URL: https://github.com/apache/servicecomb-java-chassis/pull/2157 This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org
[servicecomb-java-chassis] branch master updated: [SCB-2169] optimize filter declare and chain config
This is an automated email from the ASF dual-hosted git repository. wujimin pushed a commit to branch master in repository https://gitbox.apache.org/repos/asf/servicecomb-java-chassis.git The following commit(s) were added to refs/heads/master by this push: new 76fe676 [SCB-2169] optimize filter declare and chain config 76fe676 is described below commit 76fe676dad6d0aa12d77019246d0ea9c19eeb878 Author: wujimin AuthorDate: Tue Dec 22 10:03:16 2020 +0800 [SCB-2169] optimize filter declare and chain config --- .../rest/filter/inner/RestServerCodecFilter.java | 18 ++- .../src/test/resources/microservice.yaml | 7 +- .../org/apache/servicecomb/core/SCBEngine.java | 5 +- .../core/bootstrap/SCBEngineForTest.java | 14 +- .../core/definition/ServiceRegistryListener.java | 2 +- .../servicecomb/core/filter/ConsumerFilter.java| 16 +- .../org/apache/servicecomb/core/filter/Filter.java | 24 ++- .../core/filter/FilterChainsManager.java | 123 +--- .../servicecomb/core/filter/FilterManager.java | 162 - .../apache/servicecomb/core/filter/FilterMeta.java | 50 --- .../apache/servicecomb/core/filter/FilterNode.java | 2 +- .../{FilterProvider.java => InternalFilter.java} | 5 +- .../core/filter/InvocationFilterChains.java| 113 ++ .../servicecomb/core/filter/ProducerFilter.java| 19 ++- .../filter/config/AbstractFilterChainsConfig.java | 24 +-- .../core/filter/config/FilterChainsConfig.java | 95 +++- .../config/InvocationFilterChainsConfig.java | 69 + ...ilterConfig.java => TransportChainsConfig.java} | 14 +- .../core/filter/config/TransportFiltersConfig.java | 54 --- ...DefaultFilterProvider.java => EmptyFilter.java} | 28 ++-- .../core/filter/impl/ParameterValidatorFilter.java | 25 ++-- .../core/filter/impl/ProducerOperationFilter.java | 22 +-- .../core/filter/impl/ScheduleFilter.java | 20 ++- .../core/filter/impl/SimpleLoadBalanceFilter.java | 110 -- .../core/filter/impl/TransportFilters.java | 22 ++- core/src/main/resources/microservice.yaml | 19 ++- .../core/filter/FilterChainsManagerTest.java | 103 - .../servicecomb/core/filter/SimpleRetryFilter.java | 11 +- .../filter/impl/ParameterValidatorFilterTest.java | 2 +- .../demo/pojo/client/CodeFirstPojoClient.java | 20 +-- .../src/test/resources/microservice.yaml | 22 +-- .../src/test/resources/microservice.yaml | 22 +-- .../tracing/zipkin/ZipkinTracingFilter.java| 20 ++- .../src/test/resources/microservice.yaml | 22 +-- .../src/test/resources/microservice.yaml | 2 +- .../src/test/resources/microservice.yaml | 22 +-- .../src/test/resources/microservice.yaml | 22 +-- .../transport/highway/HighwayClientFilter.java | 27 +++- .../highway/HighwayServerCodecFilter.java | 19 ++- .../src/main}/resources/microservice.yaml | 24 +-- .../rest/client/RestClientCodecFilter.java | 20 ++- .../rest/client/RestClientFilterProvider.java | 35 - .../rest/client/RestClientSenderFilter.java| 20 ++- 43 files changed, 655 insertions(+), 820 deletions(-) diff --git a/common/common-rest/src/main/java/org/apache/servicecomb/common/rest/filter/inner/RestServerCodecFilter.java b/common/common-rest/src/main/java/org/apache/servicecomb/common/rest/filter/inner/RestServerCodecFilter.java index bcf30a5..3dd5557 100644 --- a/common/common-rest/src/main/java/org/apache/servicecomb/common/rest/filter/inner/RestServerCodecFilter.java +++ b/common/common-rest/src/main/java/org/apache/servicecomb/common/rest/filter/inner/RestServerCodecFilter.java @@ -21,12 +21,12 @@ import static com.google.common.net.HttpHeaders.CONTENT_LENGTH; import static com.google.common.net.HttpHeaders.TRANSFER_ENCODING; import static javax.ws.rs.core.Response.Status.INTERNAL_SERVER_ERROR; import static org.apache.servicecomb.core.exception.Exceptions.exceptionToResponse; -import static org.apache.servicecomb.swagger.invocation.InvocationType.PRODUCER; import java.util.Map; import java.util.Map.Entry; import java.util.concurrent.CompletableFuture; +import javax.annotation.Nonnull; import javax.servlet.http.Part; import org.apache.servicecomb.common.rest.HttpTransportContext; @@ -36,20 +36,28 @@ import org.apache.servicecomb.common.rest.codec.produce.ProduceProcessor; import org.apache.servicecomb.common.rest.definition.RestOperationMeta; import org.apache.servicecomb.core.Invocation; import org.apache.servicecomb.core.definition.OperationMeta; -import org.apache.servicecomb.core.filter.Filter; -import org.apache.servicecomb.core.filter.FilterMeta; import org.apache.servicecomb.core.filter.FilterNode; +import org.apache.servicecomb.core.filter.ProducerFilter; import