[GitHub] [commons-lang] nnivruth commented on a change in pull request #495: LANG-1519 Add zero/positive/negative util methods
nnivruth commented on a change in pull request #495: LANG-1519 Add zero/positive/negative util methods URL: https://github.com/apache/commons-lang/pull/495#discussion_r382966556 ## File path: src/main/java/org/apache/commons/lang3/math/NumberUtils.java ## @@ -1822,4 +1824,125 @@ public static int compare(final short x, final short y) { public static int compare(final byte x, final byte y) { return x - y; } + +/** + * Checks if a {@code Number} value is zero, + * handling {@code null} by returning {@code false}. + * + * @param number the {@code number} to check, {@code null} returns {@code false} + * @return {@code true} only if the input is non-null and equals zero + * @since 3.10 + */ +public static boolean isZero(final Number number) { +if (Objects.isNull(number)) { +return false; +} else if (number instanceof Integer) { +return number.intValue() == INTEGER_ZERO; +} else if (number instanceof Long) { +return number.longValue() == LONG_ZERO; +} else if (number instanceof Byte) { +return number.byteValue() == BYTE_ZERO; +} else if (number instanceof Short) { +return number.shortValue() == SHORT_ZERO; +} else if (number instanceof Float) { +return number.floatValue() == FLOAT_ZERO; +} else if (number instanceof Double) { +return number.doubleValue() == DOUBLE_ZERO; +} + +return false; Review comment: agree, updated the methods. This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org With regards, Apache Git Services
[jira] [Work logged] (LANG-1519) Add Integer Utils to library
[ https://issues.apache.org/jira/browse/LANG-1519?focusedWorklogId=391297=com.atlassian.jira.plugin.system.issuetabpanels:worklog-tabpanel#worklog-391297 ] ASF GitHub Bot logged work on LANG-1519: Author: ASF GitHub Bot Created on: 23/Feb/20 06:33 Start Date: 23/Feb/20 06:33 Worklog Time Spent: 10m Work Description: nnivruth commented on pull request #495: LANG-1519 Add zero/positive/negative util methods URL: https://github.com/apache/commons-lang/pull/495#discussion_r382966556 ## File path: src/main/java/org/apache/commons/lang3/math/NumberUtils.java ## @@ -1822,4 +1824,125 @@ public static int compare(final short x, final short y) { public static int compare(final byte x, final byte y) { return x - y; } + +/** + * Checks if a {@code Number} value is zero, + * handling {@code null} by returning {@code false}. + * + * @param number the {@code number} to check, {@code null} returns {@code false} + * @return {@code true} only if the input is non-null and equals zero + * @since 3.10 + */ +public static boolean isZero(final Number number) { +if (Objects.isNull(number)) { +return false; +} else if (number instanceof Integer) { +return number.intValue() == INTEGER_ZERO; +} else if (number instanceof Long) { +return number.longValue() == LONG_ZERO; +} else if (number instanceof Byte) { +return number.byteValue() == BYTE_ZERO; +} else if (number instanceof Short) { +return number.shortValue() == SHORT_ZERO; +} else if (number instanceof Float) { +return number.floatValue() == FLOAT_ZERO; +} else if (number instanceof Double) { +return number.doubleValue() == DOUBLE_ZERO; +} + +return false; Review comment: agree, updated the methods. This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org Issue Time Tracking --- Worklog Id: (was: 391297) Time Spent: 2h 40m (was: 2.5h) > Add Integer Utils to library > > > Key: LANG-1519 > URL: https://issues.apache.org/jira/browse/LANG-1519 > Project: Commons Lang > Issue Type: New Feature > Components: lang.* >Reporter: Nivruth Nandigam >Priority: Trivial > Time Spent: 2h 40m > Remaining Estimate: 0h > > Create Integer Utils class for useful integer operations and a placeholder > for adding more useful/important utility functions > > Pull Request: [https://github.com/apache/commons-lang/pull/495] -- This message was sent by Atlassian Jira (v8.3.4#803005)
[jira] [Work logged] (LANG-1519) Add Integer Utils to library
[ https://issues.apache.org/jira/browse/LANG-1519?focusedWorklogId=391296=com.atlassian.jira.plugin.system.issuetabpanels:worklog-tabpanel#worklog-391296 ] ASF GitHub Bot logged work on LANG-1519: Author: ASF GitHub Bot Created on: 23/Feb/20 05:46 Start Date: 23/Feb/20 05:46 Worklog Time Spent: 10m Work Description: nnivruth commented on pull request #495: LANG-1519 Add Integer Utils to library URL: https://github.com/apache/commons-lang/pull/495#discussion_r382966556 ## File path: src/main/java/org/apache/commons/lang3/math/NumberUtils.java ## @@ -1822,4 +1824,125 @@ public static int compare(final short x, final short y) { public static int compare(final byte x, final byte y) { return x - y; } + +/** + * Checks if a {@code Number} value is zero, + * handling {@code null} by returning {@code false}. + * + * @param number the {@code number} to check, {@code null} returns {@code false} + * @return {@code true} only if the input is non-null and equals zero + * @since 3.10 + */ +public static boolean isZero(final Number number) { +if (Objects.isNull(number)) { +return false; +} else if (number instanceof Integer) { +return number.intValue() == INTEGER_ZERO; +} else if (number instanceof Long) { +return number.longValue() == LONG_ZERO; +} else if (number instanceof Byte) { +return number.byteValue() == BYTE_ZERO; +} else if (number instanceof Short) { +return number.shortValue() == SHORT_ZERO; +} else if (number instanceof Float) { +return number.floatValue() == FLOAT_ZERO; +} else if (number instanceof Double) { +return number.doubleValue() == DOUBLE_ZERO; +} + +return false; Review comment: agree, updated the method. This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org Issue Time Tracking --- Worklog Id: (was: 391296) Time Spent: 2.5h (was: 2h 20m) > Add Integer Utils to library > > > Key: LANG-1519 > URL: https://issues.apache.org/jira/browse/LANG-1519 > Project: Commons Lang > Issue Type: New Feature > Components: lang.* >Reporter: Nivruth Nandigam >Priority: Trivial > Time Spent: 2.5h > Remaining Estimate: 0h > > Create Integer Utils class for useful integer operations and a placeholder > for adding more useful/important utility functions > > Pull Request: [https://github.com/apache/commons-lang/pull/495] -- This message was sent by Atlassian Jira (v8.3.4#803005)
[GitHub] [commons-lang] nnivruth commented on a change in pull request #495: LANG-1519 Add Integer Utils to library
nnivruth commented on a change in pull request #495: LANG-1519 Add Integer Utils to library URL: https://github.com/apache/commons-lang/pull/495#discussion_r382966556 ## File path: src/main/java/org/apache/commons/lang3/math/NumberUtils.java ## @@ -1822,4 +1824,125 @@ public static int compare(final short x, final short y) { public static int compare(final byte x, final byte y) { return x - y; } + +/** + * Checks if a {@code Number} value is zero, + * handling {@code null} by returning {@code false}. + * + * @param number the {@code number} to check, {@code null} returns {@code false} + * @return {@code true} only if the input is non-null and equals zero + * @since 3.10 + */ +public static boolean isZero(final Number number) { +if (Objects.isNull(number)) { +return false; +} else if (number instanceof Integer) { +return number.intValue() == INTEGER_ZERO; +} else if (number instanceof Long) { +return number.longValue() == LONG_ZERO; +} else if (number instanceof Byte) { +return number.byteValue() == BYTE_ZERO; +} else if (number instanceof Short) { +return number.shortValue() == SHORT_ZERO; +} else if (number instanceof Float) { +return number.floatValue() == FLOAT_ZERO; +} else if (number instanceof Double) { +return number.doubleValue() == DOUBLE_ZERO; +} + +return false; Review comment: agree, updated the func. This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org With regards, Apache Git Services
[jira] [Work logged] (LANG-1519) Add Integer Utils to library
[ https://issues.apache.org/jira/browse/LANG-1519?focusedWorklogId=391295=com.atlassian.jira.plugin.system.issuetabpanels:worklog-tabpanel#worklog-391295 ] ASF GitHub Bot logged work on LANG-1519: Author: ASF GitHub Bot Created on: 23/Feb/20 05:45 Start Date: 23/Feb/20 05:45 Worklog Time Spent: 10m Work Description: nnivruth commented on pull request #495: LANG-1519 Add Integer Utils to library URL: https://github.com/apache/commons-lang/pull/495#discussion_r382966556 ## File path: src/main/java/org/apache/commons/lang3/math/NumberUtils.java ## @@ -1822,4 +1824,125 @@ public static int compare(final short x, final short y) { public static int compare(final byte x, final byte y) { return x - y; } + +/** + * Checks if a {@code Number} value is zero, + * handling {@code null} by returning {@code false}. + * + * @param number the {@code number} to check, {@code null} returns {@code false} + * @return {@code true} only if the input is non-null and equals zero + * @since 3.10 + */ +public static boolean isZero(final Number number) { +if (Objects.isNull(number)) { +return false; +} else if (number instanceof Integer) { +return number.intValue() == INTEGER_ZERO; +} else if (number instanceof Long) { +return number.longValue() == LONG_ZERO; +} else if (number instanceof Byte) { +return number.byteValue() == BYTE_ZERO; +} else if (number instanceof Short) { +return number.shortValue() == SHORT_ZERO; +} else if (number instanceof Float) { +return number.floatValue() == FLOAT_ZERO; +} else if (number instanceof Double) { +return number.doubleValue() == DOUBLE_ZERO; +} + +return false; Review comment: agree, updated the func. This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org Issue Time Tracking --- Worklog Id: (was: 391295) Time Spent: 2h 20m (was: 2h 10m) > Add Integer Utils to library > > > Key: LANG-1519 > URL: https://issues.apache.org/jira/browse/LANG-1519 > Project: Commons Lang > Issue Type: New Feature > Components: lang.* >Reporter: Nivruth Nandigam >Priority: Trivial > Time Spent: 2h 20m > Remaining Estimate: 0h > > Create Integer Utils class for useful integer operations and a placeholder > for adding more useful/important utility functions > > Pull Request: [https://github.com/apache/commons-lang/pull/495] -- This message was sent by Atlassian Jira (v8.3.4#803005)
[GitHub] [commons-lang] nnivruth commented on a change in pull request #495: LANG-1519 Add Integer Utils to library
nnivruth commented on a change in pull request #495: LANG-1519 Add Integer Utils to library URL: https://github.com/apache/commons-lang/pull/495#discussion_r382966556 ## File path: src/main/java/org/apache/commons/lang3/math/NumberUtils.java ## @@ -1822,4 +1824,125 @@ public static int compare(final short x, final short y) { public static int compare(final byte x, final byte y) { return x - y; } + +/** + * Checks if a {@code Number} value is zero, + * handling {@code null} by returning {@code false}. + * + * @param number the {@code number} to check, {@code null} returns {@code false} + * @return {@code true} only if the input is non-null and equals zero + * @since 3.10 + */ +public static boolean isZero(final Number number) { +if (Objects.isNull(number)) { +return false; +} else if (number instanceof Integer) { +return number.intValue() == INTEGER_ZERO; +} else if (number instanceof Long) { +return number.longValue() == LONG_ZERO; +} else if (number instanceof Byte) { +return number.byteValue() == BYTE_ZERO; +} else if (number instanceof Short) { +return number.shortValue() == SHORT_ZERO; +} else if (number instanceof Float) { +return number.floatValue() == FLOAT_ZERO; +} else if (number instanceof Double) { +return number.doubleValue() == DOUBLE_ZERO; +} + +return false; Review comment: agree, updated the method. This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org With regards, Apache Git Services
[jira] [Work logged] (LANG-1519) Add Integer Utils to library
[ https://issues.apache.org/jira/browse/LANG-1519?focusedWorklogId=391289=com.atlassian.jira.plugin.system.issuetabpanels:worklog-tabpanel#worklog-391289 ] ASF GitHub Bot logged work on LANG-1519: Author: ASF GitHub Bot Created on: 23/Feb/20 04:45 Start Date: 23/Feb/20 04:45 Worklog Time Spent: 10m Work Description: coveralls commented on issue #495: LANG-1519 Add Integer Utils to library URL: https://github.com/apache/commons-lang/pull/495#issuecomment-589476556 [](https://coveralls.io/builds/28900868) Coverage increased (+0.004%) to 95.105% when pulling **78b691fdded3027f9be7b42de3d3430f5f59d778 on nnivruth:master** into **94b3784fdec5d0e9d63e4aec6772144b68283790 on apache:master**. This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org Issue Time Tracking --- Worklog Id: (was: 391289) Time Spent: 2h 10m (was: 2h) > Add Integer Utils to library > > > Key: LANG-1519 > URL: https://issues.apache.org/jira/browse/LANG-1519 > Project: Commons Lang > Issue Type: New Feature > Components: lang.* >Reporter: Nivruth Nandigam >Priority: Trivial > Time Spent: 2h 10m > Remaining Estimate: 0h > > Create Integer Utils class for useful integer operations and a placeholder > for adding more useful/important utility functions > > Pull Request: [https://github.com/apache/commons-lang/pull/495] -- This message was sent by Atlassian Jira (v8.3.4#803005)
[GitHub] [commons-lang] coveralls edited a comment on issue #495: LANG-1519 Add Integer Utils to library
coveralls edited a comment on issue #495: LANG-1519 Add Integer Utils to library URL: https://github.com/apache/commons-lang/pull/495#issuecomment-589476556 [](https://coveralls.io/builds/28900868) Coverage increased (+0.004%) to 95.105% when pulling **78b691fdded3027f9be7b42de3d3430f5f59d778 on nnivruth:master** into **94b3784fdec5d0e9d63e4aec6772144b68283790 on apache:master**. This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org With regards, Apache Git Services
[GitHub] [commons-csv] shootercheng commented on issue #58: export page data from db
shootercheng commented on issue #58: export page data from db URL: https://github.com/apache/commons-csv/pull/58#issuecomment-590023179 I am so sorry , I have closed it. ---Original--- From: "Gary Gregory"
[GitHub] [commons-csv] shootercheng closed pull request #58: export page data from db
shootercheng closed pull request #58: export page data from db URL: https://github.com/apache/commons-csv/pull/58 This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org With regards, Apache Git Services
[GitHub] [commons-csv] shootercheng commented on issue #58: export page data from db
shootercheng commented on issue #58: export page data from db URL: https://github.com/apache/commons-csv/pull/58#issuecomment-590022572 > > > intent > > > > > > > This ticket has no description so I have no idea what the intent is here. The lack of Javadoc does not help either. > > > > > > you can see the method DbQueryExportTest.testExport(), this test method will export querying page data from db > > @shootercheng > I'm sorry so say that you're not helping your PR here :-( You should provide some kind of description for this PR so that people can learn what problem this PR attempts to solve and how. This would then allow reviewers to try to understand if this code belongs here and how it can fit in. I suppose you could fill in the Javadoc at a later phase of your development (but before any merge into Commons CSV.) > > If you want to export database rows, you can use `org.apache.commons.csv.CSVPrinter.printRecords(ResultSet)` which works with Java's own JDBC framework. The printer class also provides other types of services to create CSV files. > > More importantly, I see zero reuse of Commons CSV's code for which this PR seems to duplicate features like using your `ExportParam` instead of CVS's `CSVFormat`. It seems this PR is complete;y standalone code from Commons CSV since it does not reuse any of Commons CSV's code. Why would it belong within Commons CSV? 之前使用过 printRecords(ResultSet) 去导出 csv 数据,但是数据量比较大的时候,导出有问题,所以我就加了这个导出方法,确实好像可以单独出来建一个工程了,不过放在这里也没啥问题呀,增强了原来的导出功能呀 This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org With regards, Apache Git Services
[GitHub] [commons-csv] garydgregory commented on issue #58: export page data from db
garydgregory commented on issue #58: export page data from db URL: https://github.com/apache/commons-csv/pull/58#issuecomment-590021088 > > intent > > > This ticket has no description so I have no idea what the intent is here. The lack of Javadoc does not help either. > > you can see the method DbQueryExportTest.testExport(), this test method will export querying page data from db @shootercheng I'm sorry so say that you're not helping your PR here :-( You should provide some kind of description for this PR so that people can learn what problem this PR attempts to solve and how. This would then allow reviewers to try to understand if this code belongs here and how it can fit in. I suppose you could fill in the Javadoc at a later phase of your development (but before any merge into Commons CSV.) If you want to export database rows, you can use `org.apache.commons.csv.CSVPrinter.printRecords(ResultSet)` which works with Java's own JDBC framework. The printer class also provides other types of services to create CSV files. More importantly, I see zero reuse of Commons CSV's code for which this PR seems to duplicate features like using your `ExportParam` instead of CVS's `CSVFormat`. It seems this PR is complete;y standalone code from Commons CSV since it does not reuse any of Commons CSV's code. Why would it belong within Commons CSV? This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org With regards, Apache Git Services
[jira] [Commented] (GEOMETRY-56) Create distribution archive
[ https://issues.apache.org/jira/browse/GEOMETRY-56?page=com.atlassian.jira.plugin.system.issuetabpanels:comment-tabpanel=17042779#comment-17042779 ] Matt Juntunen commented on GEOMETRY-56: --- Is there an example distribution project somewhere to use as a template? > Create distribution archive > --- > > Key: GEOMETRY-56 > URL: https://issues.apache.org/jira/browse/GEOMETRY-56 > Project: Apache Commons Geometry > Issue Type: Improvement >Reporter: Karl Heinz Marbaise >Assignee: Karl Heinz Marbaise >Priority: Blocker > Labels: Maven, jenkins > Fix For: 1.0 > > > *Problem* > * Currently the configuration for building a distribution archive does not > exist > * The current configuration for creating archives is wrong > *Goal* > * Create separate distribution archive module > * Create correct configuration for maven-assembly-plugin -- This message was sent by Atlassian Jira (v8.3.4#803005)
[GitHub] [commons-geometry] darkma773r opened a new pull request #68: License and Project Files
darkma773r opened a new pull request #68: License and Project Files URL: https://github.com/apache/commons-geometry/pull/68 Adding missing license and project files: - LICENSE.txt - NOTICE.txt - CONTRIBUTING.md (generated by commons-build plugin) - RELEASE-NOTES.txt (empty) This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org With regards, Apache Git Services
[GitHub] [commons-geometry] darkma773r opened a new pull request #67: License and Project Files
darkma773r opened a new pull request #67: License and Project Files URL: https://github.com/apache/commons-geometry/pull/67 Adding missing license and project files: - LICENSE.txt - NOTICE.txt - CONTRIBUTING.md (generated by commons-build plugin) - RELEASE-NOTES.txt (empty) This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org With regards, Apache Git Services
[GitHub] [commons-geometry] darkma773r commented on issue #67: License and Project Files
darkma773r commented on issue #67: License and Project Files URL: https://github.com/apache/commons-geometry/pull/67#issuecomment-590017453 Bad commit This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org With regards, Apache Git Services
[GitHub] [commons-csv] coveralls edited a comment on issue #58: export page data from db
coveralls edited a comment on issue #58: export page data from db URL: https://github.com/apache/commons-csv/pull/58#issuecomment-589916433 [](https://coveralls.io/builds/28900281) Coverage decreased (-1.3%) to 91.879% when pulling **6444993df822ece3dddca3981dc3bf323224fa6e on shootercheng:master** into **e503c568a10ae42364a94695b8505bcb4db28969 on apache:master**. This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org With regards, Apache Git Services
[GitHub] [commons-geometry] darkma773r closed pull request #67: License and Project Files
darkma773r closed pull request #67: License and Project Files URL: https://github.com/apache/commons-geometry/pull/67 This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org With regards, Apache Git Services
[GitHub] [commons-csv] shootercheng commented on issue #58: export page data from db
shootercheng commented on issue #58: export page data from db URL: https://github.com/apache/commons-csv/pull/58#issuecomment-590017392 > intent > This ticket has no description so I have no idea what the intent is here. The lack of Javadoc does not help either. you can see the method DbQueryExportTest.testExport(), this test method will export querying page data from db This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org With regards, Apache Git Services
[jira] [Work logged] (LANG-1519) Add Integer Utils to library
[ https://issues.apache.org/jira/browse/LANG-1519?focusedWorklogId=391267=com.atlassian.jira.plugin.system.issuetabpanels:worklog-tabpanel#worklog-391267 ] ASF GitHub Bot logged work on LANG-1519: Author: ASF GitHub Bot Created on: 23/Feb/20 01:19 Start Date: 23/Feb/20 01:19 Worklog Time Spent: 10m Work Description: garydgregory commented on pull request #495: LANG-1519 Add Integer Utils to library URL: https://github.com/apache/commons-lang/pull/495#discussion_r382954510 ## File path: src/main/java/org/apache/commons/lang3/math/NumberUtils.java ## @@ -1822,4 +1824,125 @@ public static int compare(final short x, final short y) { public static int compare(final byte x, final byte y) { return x - y; } + +/** + * Checks if a {@code Number} value is zero, + * handling {@code null} by returning {@code false}. + * + * @param number the {@code number} to check, {@code null} returns {@code false} + * @return {@code true} only if the input is non-null and equals zero + * @since 3.10 + */ +public static boolean isZero(final Number number) { +if (Objects.isNull(number)) { +return false; +} else if (number instanceof Integer) { +return number.intValue() == INTEGER_ZERO; +} else if (number instanceof Long) { +return number.longValue() == LONG_ZERO; +} else if (number instanceof Byte) { +return number.byteValue() == BYTE_ZERO; +} else if (number instanceof Short) { +return number.shortValue() == SHORT_ZERO; +} else if (number instanceof Float) { +return number.floatValue() == FLOAT_ZERO; +} else if (number instanceof Double) { +return number.doubleValue() == DOUBLE_ZERO; +} + +return false; Review comment: This is wrong IMO, if I create a subclass of `Number`, this method will always return false even if my `Number`'s intValue() returns 0. This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org Issue Time Tracking --- Worklog Id: (was: 391267) Time Spent: 2h (was: 1h 50m) > Add Integer Utils to library > > > Key: LANG-1519 > URL: https://issues.apache.org/jira/browse/LANG-1519 > Project: Commons Lang > Issue Type: New Feature > Components: lang.* >Reporter: Nivruth Nandigam >Priority: Trivial > Time Spent: 2h > Remaining Estimate: 0h > > Create Integer Utils class for useful integer operations and a placeholder > for adding more useful/important utility functions > > Pull Request: [https://github.com/apache/commons-lang/pull/495] -- This message was sent by Atlassian Jira (v8.3.4#803005)
[GitHub] [commons-lang] garydgregory commented on a change in pull request #495: LANG-1519 Add Integer Utils to library
garydgregory commented on a change in pull request #495: LANG-1519 Add Integer Utils to library URL: https://github.com/apache/commons-lang/pull/495#discussion_r382954510 ## File path: src/main/java/org/apache/commons/lang3/math/NumberUtils.java ## @@ -1822,4 +1824,125 @@ public static int compare(final short x, final short y) { public static int compare(final byte x, final byte y) { return x - y; } + +/** + * Checks if a {@code Number} value is zero, + * handling {@code null} by returning {@code false}. + * + * @param number the {@code number} to check, {@code null} returns {@code false} + * @return {@code true} only if the input is non-null and equals zero + * @since 3.10 + */ +public static boolean isZero(final Number number) { +if (Objects.isNull(number)) { +return false; +} else if (number instanceof Integer) { +return number.intValue() == INTEGER_ZERO; +} else if (number instanceof Long) { +return number.longValue() == LONG_ZERO; +} else if (number instanceof Byte) { +return number.byteValue() == BYTE_ZERO; +} else if (number instanceof Short) { +return number.shortValue() == SHORT_ZERO; +} else if (number instanceof Float) { +return number.floatValue() == FLOAT_ZERO; +} else if (number instanceof Double) { +return number.doubleValue() == DOUBLE_ZERO; +} + +return false; Review comment: This is wrong IMO, if I create a subclass of `Number`, this method will always return false even if my `Number`'s intValue() returns 0. This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org With regards, Apache Git Services
[jira] [Work logged] (LANG-1519) Add Integer Utils to library
[ https://issues.apache.org/jira/browse/LANG-1519?focusedWorklogId=391266=com.atlassian.jira.plugin.system.issuetabpanels:worklog-tabpanel#worklog-391266 ] ASF GitHub Bot logged work on LANG-1519: Author: ASF GitHub Bot Created on: 23/Feb/20 01:13 Start Date: 23/Feb/20 01:13 Worklog Time Spent: 10m Work Description: garydgregory commented on pull request #495: LANG-1519 Add Integer Utils to library URL: https://github.com/apache/commons-lang/pull/495#discussion_r382954510 ## File path: src/main/java/org/apache/commons/lang3/math/NumberUtils.java ## @@ -1822,4 +1824,125 @@ public static int compare(final short x, final short y) { public static int compare(final byte x, final byte y) { return x - y; } + +/** + * Checks if a {@code Number} value is zero, + * handling {@code null} by returning {@code false}. + * + * @param number the {@code number} to check, {@code null} returns {@code false} + * @return {@code true} only if the input is non-null and equals zero + * @since 3.10 + */ +public static boolean isZero(final Number number) { +if (Objects.isNull(number)) { +return false; +} else if (number instanceof Integer) { +return number.intValue() == INTEGER_ZERO; +} else if (number instanceof Long) { +return number.longValue() == LONG_ZERO; +} else if (number instanceof Byte) { +return number.byteValue() == BYTE_ZERO; +} else if (number instanceof Short) { +return number.shortValue() == SHORT_ZERO; +} else if (number instanceof Float) { +return number.floatValue() == FLOAT_ZERO; +} else if (number instanceof Double) { +return number.doubleValue() == DOUBLE_ZERO; +} + +return false; Review comment: This is bogus IMO, if I create a subclass of `Number`, this method will always return false even if my `Number`'s intValue() returns 0. This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org Issue Time Tracking --- Worklog Id: (was: 391266) Time Spent: 1h 50m (was: 1h 40m) > Add Integer Utils to library > > > Key: LANG-1519 > URL: https://issues.apache.org/jira/browse/LANG-1519 > Project: Commons Lang > Issue Type: New Feature > Components: lang.* >Reporter: Nivruth Nandigam >Priority: Trivial > Time Spent: 1h 50m > Remaining Estimate: 0h > > Create Integer Utils class for useful integer operations and a placeholder > for adding more useful/important utility functions > > Pull Request: [https://github.com/apache/commons-lang/pull/495] -- This message was sent by Atlassian Jira (v8.3.4#803005)
[GitHub] [commons-lang] garydgregory commented on a change in pull request #495: LANG-1519 Add Integer Utils to library
garydgregory commented on a change in pull request #495: LANG-1519 Add Integer Utils to library URL: https://github.com/apache/commons-lang/pull/495#discussion_r382954510 ## File path: src/main/java/org/apache/commons/lang3/math/NumberUtils.java ## @@ -1822,4 +1824,125 @@ public static int compare(final short x, final short y) { public static int compare(final byte x, final byte y) { return x - y; } + +/** + * Checks if a {@code Number} value is zero, + * handling {@code null} by returning {@code false}. + * + * @param number the {@code number} to check, {@code null} returns {@code false} + * @return {@code true} only if the input is non-null and equals zero + * @since 3.10 + */ +public static boolean isZero(final Number number) { +if (Objects.isNull(number)) { +return false; +} else if (number instanceof Integer) { +return number.intValue() == INTEGER_ZERO; +} else if (number instanceof Long) { +return number.longValue() == LONG_ZERO; +} else if (number instanceof Byte) { +return number.byteValue() == BYTE_ZERO; +} else if (number instanceof Short) { +return number.shortValue() == SHORT_ZERO; +} else if (number instanceof Float) { +return number.floatValue() == FLOAT_ZERO; +} else if (number instanceof Double) { +return number.doubleValue() == DOUBLE_ZERO; +} + +return false; Review comment: This is bogus IMO, if I create a subclass of `Number`, this method will always return false even if my `Number`'s intValue() returns 0. Why is this whole method's implementation not simply `return number.doubleValue() == DOUBLE_ZERO` ? This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org With regards, Apache Git Services
[GitHub] [commons-lang] garydgregory commented on a change in pull request #495: LANG-1519 Add Integer Utils to library
garydgregory commented on a change in pull request #495: LANG-1519 Add Integer Utils to library URL: https://github.com/apache/commons-lang/pull/495#discussion_r382954510 ## File path: src/main/java/org/apache/commons/lang3/math/NumberUtils.java ## @@ -1822,4 +1824,125 @@ public static int compare(final short x, final short y) { public static int compare(final byte x, final byte y) { return x - y; } + +/** + * Checks if a {@code Number} value is zero, + * handling {@code null} by returning {@code false}. + * + * @param number the {@code number} to check, {@code null} returns {@code false} + * @return {@code true} only if the input is non-null and equals zero + * @since 3.10 + */ +public static boolean isZero(final Number number) { +if (Objects.isNull(number)) { +return false; +} else if (number instanceof Integer) { +return number.intValue() == INTEGER_ZERO; +} else if (number instanceof Long) { +return number.longValue() == LONG_ZERO; +} else if (number instanceof Byte) { +return number.byteValue() == BYTE_ZERO; +} else if (number instanceof Short) { +return number.shortValue() == SHORT_ZERO; +} else if (number instanceof Float) { +return number.floatValue() == FLOAT_ZERO; +} else if (number instanceof Double) { +return number.doubleValue() == DOUBLE_ZERO; +} + +return false; Review comment: This is bogus IMO, if I create a subclass of `Number`, this method will always return false even if my `Number`'s intValue() returns 0. This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org With regards, Apache Git Services
[jira] [Work logged] (LANG-1519) Add Integer Utils to library
[ https://issues.apache.org/jira/browse/LANG-1519?focusedWorklogId=391264=com.atlassian.jira.plugin.system.issuetabpanels:worklog-tabpanel#worklog-391264 ] ASF GitHub Bot logged work on LANG-1519: Author: ASF GitHub Bot Created on: 23/Feb/20 01:12 Start Date: 23/Feb/20 01:12 Worklog Time Spent: 10m Work Description: garydgregory commented on pull request #495: LANG-1519 Add Integer Utils to library URL: https://github.com/apache/commons-lang/pull/495#discussion_r382954510 ## File path: src/main/java/org/apache/commons/lang3/math/NumberUtils.java ## @@ -1822,4 +1824,125 @@ public static int compare(final short x, final short y) { public static int compare(final byte x, final byte y) { return x - y; } + +/** + * Checks if a {@code Number} value is zero, + * handling {@code null} by returning {@code false}. + * + * @param number the {@code number} to check, {@code null} returns {@code false} + * @return {@code true} only if the input is non-null and equals zero + * @since 3.10 + */ +public static boolean isZero(final Number number) { +if (Objects.isNull(number)) { +return false; +} else if (number instanceof Integer) { +return number.intValue() == INTEGER_ZERO; +} else if (number instanceof Long) { +return number.longValue() == LONG_ZERO; +} else if (number instanceof Byte) { +return number.byteValue() == BYTE_ZERO; +} else if (number instanceof Short) { +return number.shortValue() == SHORT_ZERO; +} else if (number instanceof Float) { +return number.floatValue() == FLOAT_ZERO; +} else if (number instanceof Double) { +return number.doubleValue() == DOUBLE_ZERO; +} + +return false; Review comment: This is bogus IMO, if I create a subclass of `Number`, this method will always return false even if my `Number`'s inValue() returns 0. Why is this whole method's implementation not simply `return number.doubleValue() == DOUBLE_ZERO` ? This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org Issue Time Tracking --- Worklog Id: (was: 391264) Time Spent: 1.5h (was: 1h 20m) > Add Integer Utils to library > > > Key: LANG-1519 > URL: https://issues.apache.org/jira/browse/LANG-1519 > Project: Commons Lang > Issue Type: New Feature > Components: lang.* >Reporter: Nivruth Nandigam >Priority: Trivial > Time Spent: 1.5h > Remaining Estimate: 0h > > Create Integer Utils class for useful integer operations and a placeholder > for adding more useful/important utility functions > > Pull Request: [https://github.com/apache/commons-lang/pull/495] -- This message was sent by Atlassian Jira (v8.3.4#803005)
[jira] [Work logged] (LANG-1519) Add Integer Utils to library
[ https://issues.apache.org/jira/browse/LANG-1519?focusedWorklogId=391265=com.atlassian.jira.plugin.system.issuetabpanels:worklog-tabpanel#worklog-391265 ] ASF GitHub Bot logged work on LANG-1519: Author: ASF GitHub Bot Created on: 23/Feb/20 01:12 Start Date: 23/Feb/20 01:12 Worklog Time Spent: 10m Work Description: garydgregory commented on pull request #495: LANG-1519 Add Integer Utils to library URL: https://github.com/apache/commons-lang/pull/495#discussion_r382954510 ## File path: src/main/java/org/apache/commons/lang3/math/NumberUtils.java ## @@ -1822,4 +1824,125 @@ public static int compare(final short x, final short y) { public static int compare(final byte x, final byte y) { return x - y; } + +/** + * Checks if a {@code Number} value is zero, + * handling {@code null} by returning {@code false}. + * + * @param number the {@code number} to check, {@code null} returns {@code false} + * @return {@code true} only if the input is non-null and equals zero + * @since 3.10 + */ +public static boolean isZero(final Number number) { +if (Objects.isNull(number)) { +return false; +} else if (number instanceof Integer) { +return number.intValue() == INTEGER_ZERO; +} else if (number instanceof Long) { +return number.longValue() == LONG_ZERO; +} else if (number instanceof Byte) { +return number.byteValue() == BYTE_ZERO; +} else if (number instanceof Short) { +return number.shortValue() == SHORT_ZERO; +} else if (number instanceof Float) { +return number.floatValue() == FLOAT_ZERO; +} else if (number instanceof Double) { +return number.doubleValue() == DOUBLE_ZERO; +} + +return false; Review comment: This is bogus IMO, if I create a subclass of `Number`, this method will always return false even if my `Number`'s intValue() returns 0. Why is this whole method's implementation not simply `return number.doubleValue() == DOUBLE_ZERO` ? This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org Issue Time Tracking --- Worklog Id: (was: 391265) Time Spent: 1h 40m (was: 1.5h) > Add Integer Utils to library > > > Key: LANG-1519 > URL: https://issues.apache.org/jira/browse/LANG-1519 > Project: Commons Lang > Issue Type: New Feature > Components: lang.* >Reporter: Nivruth Nandigam >Priority: Trivial > Time Spent: 1h 40m > Remaining Estimate: 0h > > Create Integer Utils class for useful integer operations and a placeholder > for adding more useful/important utility functions > > Pull Request: [https://github.com/apache/commons-lang/pull/495] -- This message was sent by Atlassian Jira (v8.3.4#803005)
[GitHub] [commons-lang] garydgregory commented on a change in pull request #495: LANG-1519 Add Integer Utils to library
garydgregory commented on a change in pull request #495: LANG-1519 Add Integer Utils to library URL: https://github.com/apache/commons-lang/pull/495#discussion_r382954510 ## File path: src/main/java/org/apache/commons/lang3/math/NumberUtils.java ## @@ -1822,4 +1824,125 @@ public static int compare(final short x, final short y) { public static int compare(final byte x, final byte y) { return x - y; } + +/** + * Checks if a {@code Number} value is zero, + * handling {@code null} by returning {@code false}. + * + * @param number the {@code number} to check, {@code null} returns {@code false} + * @return {@code true} only if the input is non-null and equals zero + * @since 3.10 + */ +public static boolean isZero(final Number number) { +if (Objects.isNull(number)) { +return false; +} else if (number instanceof Integer) { +return number.intValue() == INTEGER_ZERO; +} else if (number instanceof Long) { +return number.longValue() == LONG_ZERO; +} else if (number instanceof Byte) { +return number.byteValue() == BYTE_ZERO; +} else if (number instanceof Short) { +return number.shortValue() == SHORT_ZERO; +} else if (number instanceof Float) { +return number.floatValue() == FLOAT_ZERO; +} else if (number instanceof Double) { +return number.doubleValue() == DOUBLE_ZERO; +} + +return false; Review comment: This is bogus IMO, if I create a subclass of `Number`, this method will always return false even if my `Number`'s inValue() returns 0. Why is this whole method's implementation not simply `return number.doubleValue() == DOUBLE_ZERO` ? This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org With regards, Apache Git Services
[GitHub] [commons-csv] shootercheng commented on issue #58: export page data from db
shootercheng commented on issue #58: export page data from db URL: https://github.com/apache/commons-csv/pull/58#issuecomment-590014980 今天添加JavaDoc ,实现分页查询导出数据库的数据到 CSV ---Original--- From: "Gary Gregory"
[jira] [Work logged] (LANG-1519) Add Integer Utils to library
[ https://issues.apache.org/jira/browse/LANG-1519?focusedWorklogId=391257=com.atlassian.jira.plugin.system.issuetabpanels:worklog-tabpanel#worklog-391257 ] ASF GitHub Bot logged work on LANG-1519: Author: ASF GitHub Bot Created on: 22/Feb/20 23:45 Start Date: 22/Feb/20 23:45 Worklog Time Spent: 10m Work Description: coveralls commented on issue #495: LANG-1519 Add Integer Utils to library URL: https://github.com/apache/commons-lang/pull/495#issuecomment-589476556 [](https://coveralls.io/builds/28899579) Coverage increased (+0.02%) to 95.116% when pulling **59c5ffe0f542d776a4b554067add64e5086bf9d6 on nnivruth:master** into **94b3784fdec5d0e9d63e4aec6772144b68283790 on apache:master**. This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org Issue Time Tracking --- Worklog Id: (was: 391257) Time Spent: 1h 20m (was: 1h 10m) > Add Integer Utils to library > > > Key: LANG-1519 > URL: https://issues.apache.org/jira/browse/LANG-1519 > Project: Commons Lang > Issue Type: New Feature > Components: lang.* >Reporter: Nivruth Nandigam >Priority: Trivial > Time Spent: 1h 20m > Remaining Estimate: 0h > > Create Integer Utils class for useful integer operations and a placeholder > for adding more useful/important utility functions > > Pull Request: [https://github.com/apache/commons-lang/pull/495] -- This message was sent by Atlassian Jira (v8.3.4#803005)
[GitHub] [commons-lang] coveralls edited a comment on issue #495: LANG-1519 Add Integer Utils to library
coveralls edited a comment on issue #495: LANG-1519 Add Integer Utils to library URL: https://github.com/apache/commons-lang/pull/495#issuecomment-589476556 [](https://coveralls.io/builds/28899579) Coverage increased (+0.02%) to 95.116% when pulling **59c5ffe0f542d776a4b554067add64e5086bf9d6 on nnivruth:master** into **94b3784fdec5d0e9d63e4aec6772144b68283790 on apache:master**. This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org With regards, Apache Git Services
[jira] [Work logged] (LANG-1519) Add Integer Utils to library
[ https://issues.apache.org/jira/browse/LANG-1519?focusedWorklogId=391256=com.atlassian.jira.plugin.system.issuetabpanels:worklog-tabpanel#worklog-391256 ] ASF GitHub Bot logged work on LANG-1519: Author: ASF GitHub Bot Created on: 22/Feb/20 23:38 Start Date: 22/Feb/20 23:38 Worklog Time Spent: 10m Work Description: coveralls commented on issue #495: LANG-1519 Add Integer Utils to library URL: https://github.com/apache/commons-lang/pull/495#issuecomment-589476556 [](https://coveralls.io/builds/28899542) Coverage increased (+0.008%) to 95.11% when pulling **2210d8bd901759a6a8187b349cec209804bfef1f on nnivruth:master** into **eb8d069089364e396e37ed5273cf7710e41eb06d on apache:master**. This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org Issue Time Tracking --- Worklog Id: (was: 391256) Time Spent: 1h 10m (was: 1h) > Add Integer Utils to library > > > Key: LANG-1519 > URL: https://issues.apache.org/jira/browse/LANG-1519 > Project: Commons Lang > Issue Type: New Feature > Components: lang.* >Reporter: Nivruth Nandigam >Priority: Trivial > Time Spent: 1h 10m > Remaining Estimate: 0h > > Create Integer Utils class for useful integer operations and a placeholder > for adding more useful/important utility functions > > Pull Request: [https://github.com/apache/commons-lang/pull/495] -- This message was sent by Atlassian Jira (v8.3.4#803005)
[GitHub] [commons-lang] coveralls edited a comment on issue #495: LANG-1519 Add Integer Utils to library
coveralls edited a comment on issue #495: LANG-1519 Add Integer Utils to library URL: https://github.com/apache/commons-lang/pull/495#issuecomment-589476556 [](https://coveralls.io/builds/28899542) Coverage increased (+0.008%) to 95.11% when pulling **2210d8bd901759a6a8187b349cec209804bfef1f on nnivruth:master** into **eb8d069089364e396e37ed5273cf7710e41eb06d on apache:master**. This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org With regards, Apache Git Services
[jira] [Work logged] (LANG-1519) Add Integer Utils to library
[ https://issues.apache.org/jira/browse/LANG-1519?focusedWorklogId=391254=com.atlassian.jira.plugin.system.issuetabpanels:worklog-tabpanel#worklog-391254 ] ASF GitHub Bot logged work on LANG-1519: Author: ASF GitHub Bot Created on: 22/Feb/20 23:22 Start Date: 22/Feb/20 23:22 Worklog Time Spent: 10m Work Description: nnivruth commented on issue #495: LANG-1519 Add Integer Utils to library URL: https://github.com/apache/commons-lang/pull/495#issuecomment-590008121 @ameyjadiye @garydgregory refactored & moved to NumberUtils This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org Issue Time Tracking --- Worklog Id: (was: 391254) Time Spent: 1h (was: 50m) > Add Integer Utils to library > > > Key: LANG-1519 > URL: https://issues.apache.org/jira/browse/LANG-1519 > Project: Commons Lang > Issue Type: New Feature > Components: lang.* >Reporter: Nivruth Nandigam >Priority: Trivial > Time Spent: 1h > Remaining Estimate: 0h > > Create Integer Utils class for useful integer operations and a placeholder > for adding more useful/important utility functions > > Pull Request: [https://github.com/apache/commons-lang/pull/495] -- This message was sent by Atlassian Jira (v8.3.4#803005)
[GitHub] [commons-lang] nnivruth commented on issue #495: LANG-1519 Add Integer Utils to library
nnivruth commented on issue #495: LANG-1519 Add Integer Utils to library URL: https://github.com/apache/commons-lang/pull/495#issuecomment-590008121 @ameyjadiye @garydgregory refactored & moved to NumberUtils This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org With regards, Apache Git Services
[GitHub] [commons-io] coveralls edited a comment on issue #102: Improvement of FileUtils test coverage
coveralls edited a comment on issue #102: Improvement of FileUtils test coverage URL: https://github.com/apache/commons-io/pull/102#issuecomment-590004010 [](https://coveralls.io/builds/28899213) Coverage increased (+0.1%) to 89.587% when pulling **f26380ab57a182fd27bfcf55eb92540ed6962343 on Kled9:improvement-of-fileutils-test-coverage** into **11f0abe7a3fb6954b2985ca4ab0697b2fb489e84 on apache:master**. This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org With regards, Apache Git Services
[GitHub] [commons-io] coveralls commented on issue #102: Improvement of FileUtils test coverage
coveralls commented on issue #102: Improvement of FileUtils test coverage URL: https://github.com/apache/commons-io/pull/102#issuecomment-590004010 [](https://coveralls.io/builds/28899188) Coverage increased (+0.07%) to 89.538% when pulling **f26380ab57a182fd27bfcf55eb92540ed6962343 on Kled9:improvement-of-fileutils-test-coverage** into **11f0abe7a3fb6954b2985ca4ab0697b2fb489e84 on apache:master**. This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org With regards, Apache Git Services
[GitHub] [commons-io] Kled9 opened a new pull request #102: Improvement of FileUtils test coverage
Kled9 opened a new pull request #102: Improvement of FileUtils test coverage URL: https://github.com/apache/commons-io/pull/102 This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org With regards, Apache Git Services
[GitHub] [commons-io] Kled9 closed pull request #75: Addition of tests for FileUtils
Kled9 closed pull request #75: Addition of tests for FileUtils URL: https://github.com/apache/commons-io/pull/75 This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org With regards, Apache Git Services
[GitHub] [commons-math] PRITI1999 opened a new pull request #119: Annotated FastMath.java
PRITI1999 opened a new pull request #119: Annotated FastMath.java URL: https://github.com/apache/commons-math/pull/119 This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org With regards, Apache Git Services
[GitHub] [commons-math] PRITI1999 closed pull request #119: Annotated FastMath.java
PRITI1999 closed pull request #119: Annotated FastMath.java URL: https://github.com/apache/commons-math/pull/119 This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org With regards, Apache Git Services
[GitHub] [commons-geometry] darkma773r commented on issue #66: GEOMETRY-89: Normal Transforms
darkma773r commented on issue #66: GEOMETRY-89: Normal Transforms URL: https://github.com/apache/commons-geometry/pull/66#issuecomment-589978515 First commit had a javadoc error in JDK 13. I amended the original commit with the fix. This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org With regards, Apache Git Services
[GitHub] [commons-vfs] XenoAmess commented on issue #87: Fix travis-ci to make allow_failures: - openjdk-ea due to https://jira.apache.org/jira/browse/VFS-765
XenoAmess commented on issue #87: Fix travis-ci to make allow_failures: - openjdk-ea due to https://jira.apache.org/jira/browse/VFS-765 URL: https://github.com/apache/commons-vfs/pull/87#issuecomment-589977823 > BTW if you notice such a thing, don't complain about month of people not noticing, just open a bug, this also makes changelog nicer. sorry for being rude. > I suspect this is a dependency problem, have your actually checked if it can be fixed, instead? Nope I can't. I use jdk-15 ea on windows to run this project, and cannot get this error. In other words jackrabit1 can pass build on my PC. This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org With regards, Apache Git Services
[jira] [Resolved] (VFS-764) [webdav][tests] testing fails on java 15-ea with travis
[ https://issues.apache.org/jira/browse/VFS-764?page=com.atlassian.jira.plugin.system.issuetabpanels:all-tabpanel ] Bernd Eckenfels resolved VFS-764. - Resolution: Duplicate > [webdav][tests] testing fails on java 15-ea with travis > --- > > Key: VFS-764 > URL: https://issues.apache.org/jira/browse/VFS-764 > Project: Commons VFS > Issue Type: Bug >Reporter: Bernd Eckenfels >Priority: Major > -- This message was sent by Atlassian Jira (v8.3.4#803005)
[jira] [Commented] (VFS-700) Some tests fail on Java 11 and above
[ https://issues.apache.org/jira/browse/VFS-700?page=com.atlassian.jira.plugin.system.issuetabpanels:comment-tabpanel=17042639#comment-17042639 ] Bernd Eckenfels commented on VFS-700: - This does not fail anymore, not sure if by accident or intentional, the system property is set. i will leave that open till the certificate ismchecked/renewed and the property is therefore removed. > Some tests fail on Java 11 and above > > > Key: VFS-700 > URL: https://issues.apache.org/jira/browse/VFS-700 > Project: Commons VFS > Issue Type: Bug >Reporter: Gary D. Gregory >Priority: Major > > Some tests fail on Java 11 and above: https://travis-ci.org/apache/commons-vfs > {noformat} > Tests in error: > > AbstractFtpsProviderTestCase$FtpProviderTestSuite>AbstractTestSuite.run:137->setUp:153->AbstractTestSuite.setUp:166 > » FileSystem > > AbstractFtpsProviderTestCase$FtpProviderTestSuite>AbstractTestSuite.run:137->setUp:153->AbstractTestSuite.setUp:166 > » FileSystem > MultipleConnectionTestCase.testConnectRoot:55->resolveRoot:49 » FileSystem > Cou... > MultipleConnectionTestCase.testUnderlyingConnect:65 » SSL Unsupported or > unrec... > {noformat} -- This message was sent by Atlassian Jira (v8.3.4#803005)
[jira] [Commented] (GEOMETRY-89) Normal Transforms
[ https://issues.apache.org/jira/browse/GEOMETRY-89?page=com.atlassian.jira.plugin.system.issuetabpanels:comment-tabpanel=17042636#comment-17042636 ] Matt Juntunen commented on GEOMETRY-89: --- PR is available: https://github.com/apache/commons-geometry/pull/66 > Normal Transforms > - > > Key: GEOMETRY-89 > URL: https://issues.apache.org/jira/browse/GEOMETRY-89 > Project: Apache Commons Geometry > Issue Type: New Feature >Reporter: Matt Juntunen >Priority: Major > Labels: pull-request-available > Time Spent: 10m > Remaining Estimate: 0h > > Add methods to {{AffineTransformMatrixXD}} to support creation of normal > transforms, as described > [here|https://en.wikipedia.org/wiki/Normal_(geometry)#Transforming_normals]. -- This message was sent by Atlassian Jira (v8.3.4#803005)
[jira] [Updated] (GEOMETRY-89) Normal Transforms
[ https://issues.apache.org/jira/browse/GEOMETRY-89?page=com.atlassian.jira.plugin.system.issuetabpanels:all-tabpanel ] ASF GitHub Bot updated GEOMETRY-89: --- Labels: pull-request-available (was: ) > Normal Transforms > - > > Key: GEOMETRY-89 > URL: https://issues.apache.org/jira/browse/GEOMETRY-89 > Project: Apache Commons Geometry > Issue Type: New Feature >Reporter: Matt Juntunen >Priority: Major > Labels: pull-request-available > > Add methods to {{AffineTransformMatrixXD}} to support creation of normal > transforms, as described > [here|https://en.wikipedia.org/wiki/Normal_(geometry)#Transforming_normals]. -- This message was sent by Atlassian Jira (v8.3.4#803005)
[GitHub] [commons-geometry] darkma773r opened a new pull request #66: GEOMETRY-89: Normal Transforms
darkma773r opened a new pull request #66: GEOMETRY-89: Normal Transforms URL: https://github.com/apache/commons-geometry/pull/66 Adding methods related to computation of normal transforms to AffineTransformMatrixXD. This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org With regards, Apache Git Services
[GitHub] [commons-vfs] ecki commented on issue #87: Fix travis-ci to make allow_failures: - openjdk-ea
ecki commented on issue #87: Fix travis-ci to make allow_failures: - openjdk-ea URL: https://github.com/apache/commons-vfs/pull/87#issuecomment-589975438 > I suspect this is a dependency problem, have your actually checked if it can be fixed, instead? Hm ok, is a WebDAV Test failure. I created https://jira.apache.org/jira/browse/VFS-765 - maybe you can add this to your commit message? This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org With regards, Apache Git Services
[jira] [Commented] (GEOMETRY-89) Normal Transforms
[ https://issues.apache.org/jira/browse/GEOMETRY-89?page=com.atlassian.jira.plugin.system.issuetabpanels:comment-tabpanel=17042628#comment-17042628 ] Matt Juntunen commented on GEOMETRY-89: --- I needed this functionality in my day job and thought it should be added here. > Normal Transforms > - > > Key: GEOMETRY-89 > URL: https://issues.apache.org/jira/browse/GEOMETRY-89 > Project: Apache Commons Geometry > Issue Type: New Feature >Reporter: Matt Juntunen >Priority: Major > > Add methods to {{AffineTransformMatrixXD}} to support creation of normal > transforms, as described > [here|https://en.wikipedia.org/wiki/Normal_(geometry)#Transforming_normals]. -- This message was sent by Atlassian Jira (v8.3.4#803005)
[jira] [Created] (VFS-765) [webdav][tests] jackrabit1 module webdav tests fail with IllegalStateException on java 15-ea
Bernd Eckenfels created VFS-765: --- Summary: [webdav][tests] jackrabit1 module webdav tests fail with IllegalStateException on java 15-ea Key: VFS-765 URL: https://issues.apache.org/jira/browse/VFS-765 Project: Commons VFS Issue Type: Bug Affects Versions: 2.6.0 Reporter: Bernd Eckenfels The tests of the kackrabit1 module fail on Travis-CI when using java 15-ea builds: {{Running org.apache.commons.vfs2.provider.webdav.test.WebdavProviderTestCase}} {{ 4826SLF4J: Class path contains multiple SLF4J bindings.}} {{ 4827SLF4J: Found binding in [jar:file:/home/travis/.m2/repository/org/slf4j/slf4j-log4j12/1.5.11/slf4j-log4j12-1.5.11.jar!/org/slf4j/impl/StaticLoggerBinder.class]}} {{ 4828SLF4J: Found binding in [jar:file:/home/travis/.m2/repository/org/apache/jackrabbit/jackrabbit-standalone/1.6.5/jackrabbit-standalone-1.6.5.jar!/org/slf4j/impl/StaticLoggerBinder.class]}} {{ 4829SLF4J: See [http://www.slf4j.org/codes.html#multiple_bindings] for an explanation.}} {{ 4830Tests run: 1, Failures: 0, Errors: 1, Skipped: 0, Time elapsed: 4.282 sec <<< FAILURE! - in org.apache.commons.vfs2.provider.webdav.test.WebdavProviderTestCase}} {{ 4831junit.framework.TestSuite@5c73f672(org.apache.commons.vfs2.provider.webdav.test.WebdavProviderTestCase$1) Time elapsed: 4.282 sec <<< ERROR!}} {{ 4832java.lang.IllegalStateException: Not initialized}} {{ 4833 at org.apache.commons.vfs2.provider.webdav.test.WebdavProviderTestCase$1.setUp(WebdavProviderTestCase.java:244)}} {{This is fixed by https://github.com/apache/commons-vfs/pull/87}} -- This message was sent by Atlassian Jira (v8.3.4#803005)
[jira] [Created] (VFS-764) [webdav][tests] testing fails on java 15-ea with travis
Bernd Eckenfels created VFS-764: --- Summary: [webdav][tests] testing fails on java 15-ea with travis Key: VFS-764 URL: https://issues.apache.org/jira/browse/VFS-764 Project: Commons VFS Issue Type: Bug Reporter: Bernd Eckenfels -- This message was sent by Atlassian Jira (v8.3.4#803005)
[jira] [Created] (GEOMETRY-89) Normal Transforms
Matt Juntunen created GEOMETRY-89: - Summary: Normal Transforms Key: GEOMETRY-89 URL: https://issues.apache.org/jira/browse/GEOMETRY-89 Project: Apache Commons Geometry Issue Type: New Feature Reporter: Matt Juntunen Add methods to {{AffineTransformMatrixXD}} to support creation of normal transforms, as described [here|https://en.wikipedia.org/wiki/Normal_(geometry)#Transforming_normals]. -- This message was sent by Atlassian Jira (v8.3.4#803005)
[GitHub] [commons-vfs] ecki commented on issue #87: Fix travis-ci to make allow_failures: - openjdk-ea
ecki commented on issue #87: Fix travis-ci to make allow_failures: - openjdk-ea URL: https://github.com/apache/commons-vfs/pull/87#issuecomment-589973445 Thanks, will merge it when the builds succeed. BTW if you notice such a thing, don't complain about month of people not noticing, just open a bug, this also makes changelog nicer. I suspect this is a dependency problem, have your actually checked if it can be fixed, instead? This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org With regards, Apache Git Services
[GitHub] [commons-vfs] XenoAmess opened a new pull request #87: Fix travis-ci to make allow_failures: - openjdk-ea
XenoAmess opened a new pull request #87: Fix travis-ci to make allow_failures: - openjdk-ea URL: https://github.com/apache/commons-vfs/pull/87 this project cannot pass travis since Jul 22, 2019. If you do care about the error on openjdk-ea, you shall fix it. If you do not care, then shall add openjdk-ea to allow_failures. It is not recommended to keep project failing travis for such a long time (several months till today.) This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org With regards, Apache Git Services
[GitHub] [commons-vfs] XenoAmess closed pull request #86: Fix travis-ci to make allow_failures: - openjdk-ea
XenoAmess closed pull request #86: Fix travis-ci to make allow_failures: - openjdk-ea URL: https://github.com/apache/commons-vfs/pull/86 This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org With regards, Apache Git Services
[GitHub] [commons-vfs] XenoAmess opened a new pull request #86: Fix travis-ci to make allow_failures: - openjdk-ea
XenoAmess opened a new pull request #86: Fix travis-ci to make allow_failures: - openjdk-ea URL: https://github.com/apache/commons-vfs/pull/86 this project cannot pass travis since Jul 22, 2019. If you do care about the error on openjdk-ea, you shall fix it. If you do not care, then shall add openjdk-ea to allow_failures. It is not recommended to keep project failing travis for such a long time (several months till today.) This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org With regards, Apache Git Services
[GitHub] [commons-vfs] XenoAmess closed pull request #86: Fix travis-ci to make allow_failures: - openjdk-ea
XenoAmess closed pull request #86: Fix travis-ci to make allow_failures: - openjdk-ea URL: https://github.com/apache/commons-vfs/pull/86 This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org With regards, Apache Git Services
[GitHub] [commons-vfs] XenoAmess opened a new pull request #86: Fix travis-ci to make allow_failures: - openjdk-ea
XenoAmess opened a new pull request #86: Fix travis-ci to make allow_failures: - openjdk-ea URL: https://github.com/apache/commons-vfs/pull/86 this project cannot pass travis since Jul 22, 2019. If you do care about the error on openjdk-ea, you shall fix it. If you do not care, then shall add openjdk-ea to allow_failures. It is not recommended to keep project failing travis for such a long time (several months till today.) This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org With regards, Apache Git Services
[jira] [Work logged] (LANG-341) Add number to byte array methods
[ https://issues.apache.org/jira/browse/LANG-341?focusedWorklogId=391113=com.atlassian.jira.plugin.system.issuetabpanels:worklog-tabpanel#worklog-391113 ] ASF GitHub Bot logged work on LANG-341: --- Author: ASF GitHub Bot Created on: 22/Feb/20 13:52 Start Date: 22/Feb/20 13:52 Worklog Time Spent: 10m Work Description: garydgregory commented on issue #219: LANG-341: Add number to byte array methods to org.apache.commons.lang3.Conversion URL: https://github.com/apache/commons-lang/pull/219#issuecomment-589958248 Needs conflicts resolved... This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org Issue Time Tracking --- Worklog Id: (was: 391113) Remaining Estimate: 0h Time Spent: 10m > Add number to byte array methods > > > Key: LANG-341 > URL: https://issues.apache.org/jira/browse/LANG-341 > Project: Commons Lang > Issue Type: New Feature > Components: lang.* >Reporter: Lilianne E. Blaze >Priority: Major > Fix For: Discussion > > Attachments: 341-v1-src.patch, 341-v1-test.patch, LANG-341-2.patch, > LANG-341.patch > > Time Spent: 10m > Remaining Estimate: 0h > > Hello, > I need a set of methods to convert Long to or from a byte[] array, as if > writing / reading from Data(Input/Output)Stream( > ByteArray(Input/Output)Stream ). > First, doing it with Streams seems a bit wasteful, second, it seems a > pretty general use. Would it be possible to add something like that to, > for example, > org.apache.commons.lang.math.NumberUtils? > Example code: > {code:java} > static public long toLong(byte[] b) > { > return toLong(b, 0); > } > > static public long toLong(byte[] b, int offset) > { > return (((long)b[offset] << 56) + > ((long)(b[offset + 1] & 255) << 48) + > ((long)(b[offset + 2] & 255) << 40) + > ((long)(b[offset + 3] & 255) << 32) + > ((long)(b[offset + 4] & 255) << 24) + > ((b[offset + 5] & 255) << 16) + > ((b[offset + 6] & 255) << 8) + > ((b[offset + 7] & 255) << 0)); > } > > static public byte[] longToByteArray(long l) > { > byte b[] = new byte[8]; > longToByteArray(l, b, 0); > return b; > } > > static public void longToByteArray(long l, byte b[], int offset) > { > b[offset] = (byte)(l >>> 56); > b[offset + 1] = (byte)(l >>> 48); > b[offset + 2] = (byte)(l >>> 40); > b[offset + 3] = (byte)(l >>> 32); > b[offset + 4] = (byte)(l >>> 24); > b[offset + 5] = (byte)(l >>> 16); > b[offset + 6] = (byte)(l >>> 8); > b[offset + 7] = (byte)(l >>> 0); > } > {code} -- This message was sent by Atlassian Jira (v8.3.4#803005)
[GitHub] [commons-lang] garydgregory commented on issue #219: LANG-341: Add number to byte array methods to org.apache.commons.lang3.Conversion
garydgregory commented on issue #219: LANG-341: Add number to byte array methods to org.apache.commons.lang3.Conversion URL: https://github.com/apache/commons-lang/pull/219#issuecomment-589958248 Needs conflicts resolved... This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org With regards, Apache Git Services
[jira] [Work logged] (LANG-1406) StringIndexOutOfBoundsException in StringUtils.replaceIgnoreCase()
[ https://issues.apache.org/jira/browse/LANG-1406?focusedWorklogId=391112=com.atlassian.jira.plugin.system.issuetabpanels:worklog-tabpanel#worklog-391112 ] ASF GitHub Bot logged work on LANG-1406: Author: ASF GitHub Bot Created on: 22/Feb/20 13:51 Start Date: 22/Feb/20 13:51 Worklog Time Spent: 10m Work Description: garydgregory commented on issue #381: LANG-1406 StringIndexOutOfBoundsException in StringUtils.replaceIgnor… URL: https://github.com/apache/commons-lang/pull/381#issuecomment-589958166 Needs conflicts resolved... This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org Issue Time Tracking --- Worklog Id: (was: 391112) Time Spent: 3h 20m (was: 3h 10m) > StringIndexOutOfBoundsException in StringUtils.replaceIgnoreCase() > -- > > Key: LANG-1406 > URL: https://issues.apache.org/jira/browse/LANG-1406 > Project: Commons Lang > Issue Type: Bug > Components: lang.* >Reporter: Michael Ryan >Priority: Major > Fix For: 3.10 > > Time Spent: 3h 20m > Remaining Estimate: 0h > > STEPS TO REPRODUCE: > {code} > StringUtils.replaceIgnoreCase("\u0130x", "x", "") > {code} > EXPECTED: "\u0130" is returned. > ACTUAL: StringIndexOutOfBoundsException > This happens because the replace method is assuming that text.length() == > text.toLowerCase().length(), which is not true for certain characters. -- This message was sent by Atlassian Jira (v8.3.4#803005)
[GitHub] [commons-lang] garydgregory commented on issue #381: LANG-1406 StringIndexOutOfBoundsException in StringUtils.replaceIgnor…
garydgregory commented on issue #381: LANG-1406 StringIndexOutOfBoundsException in StringUtils.replaceIgnor… URL: https://github.com/apache/commons-lang/pull/381#issuecomment-589958166 Needs conflicts resolved... This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org With regards, Apache Git Services
[jira] [Work logged] (LANG-1484) NumberUtils.isParsable returns false for strings ending with .
[ https://issues.apache.org/jira/browse/LANG-1484?focusedWorklogId=39=com.atlassian.jira.plugin.system.issuetabpanels:worklog-tabpanel#worklog-39 ] ASF GitHub Bot logged work on LANG-1484: Author: ASF GitHub Bot Created on: 22/Feb/20 13:48 Start Date: 22/Feb/20 13:48 Worklog Time Spent: 10m Work Description: garydgregory commented on issue #455: LANG-1484: Allow decimal point at the end of the String URL: https://github.com/apache/commons-lang/pull/455#issuecomment-589957886 Not a fan unless `testIsParsable` is updated to actually prove that something is parsable or not; for example, update: ``` assertTrue( NumberUtils.isParsable("64") ); ``` to ``` assertTrue( NumberUtils.isParsable("64") ); Integer.parseInt("64"); ``` This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org Issue Time Tracking --- Worklog Id: (was: 39) Time Spent: 1.5h (was: 1h 20m) > NumberUtils.isParsable returns false for strings ending with . > -- > > Key: LANG-1484 > URL: https://issues.apache.org/jira/browse/LANG-1484 > Project: Commons Lang > Issue Type: Bug > Components: lang.math.* >Affects Versions: 3.8.1 > Environment: Tested on Linux with OpenJDK 10.0.2 and Windows with > Oracle Java 8 Update 201. >Reporter: Kyle Dodson >Priority: Minor > Time Spent: 1.5h > Remaining Estimate: 0h > > From the Javadocs: > {quote}Parsable numbers include those Strings understood by ... > Double.parseDouble(String). > {quote} > {{Double.parseDouble("100.")}} returns a valid double (it does not throw a > {{NumberFormatException}}); however, {{NumberUtils.isParsable("100.")}} > returns {{false}}. > -- This message was sent by Atlassian Jira (v8.3.4#803005)
[GitHub] [commons-lang] garydgregory commented on issue #455: LANG-1484: Allow decimal point at the end of the String
garydgregory commented on issue #455: LANG-1484: Allow decimal point at the end of the String URL: https://github.com/apache/commons-lang/pull/455#issuecomment-589957886 Not a fan unless `testIsParsable` is updated to actually prove that something is parsable or not; for example, update: ``` assertTrue( NumberUtils.isParsable("64") ); ``` to ``` assertTrue( NumberUtils.isParsable("64") ); Integer.parseInt("64"); ``` This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org With regards, Apache Git Services
[GitHub] [commons-lang] garydgregory commented on issue #482: LANG-1504 - Adding steps feature to StopWatch
garydgregory commented on issue #482: LANG-1504 - Adding steps feature to StopWatch URL: https://github.com/apache/commons-lang/pull/482#issuecomment-589957106 Let's have this PR's conflicts resolved, rename "steps" to "splits" to match the current terminology and then we can have another look. This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org With regards, Apache Git Services
[jira] [Work logged] (LANG-1504) StopWatch: steps feature
[ https://issues.apache.org/jira/browse/LANG-1504?focusedWorklogId=391108=com.atlassian.jira.plugin.system.issuetabpanels:worklog-tabpanel#worklog-391108 ] ASF GitHub Bot logged work on LANG-1504: Author: ASF GitHub Bot Created on: 22/Feb/20 13:38 Start Date: 22/Feb/20 13:38 Worklog Time Spent: 10m Work Description: garydgregory commented on issue #482: LANG-1504 - Adding steps feature to StopWatch URL: https://github.com/apache/commons-lang/pull/482#issuecomment-589957106 Let's have this PR's conflicts resolved, rename "steps" to "splits" to match the current terminology and then we can have another look. This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org Issue Time Tracking --- Worklog Id: (was: 391108) Time Spent: 1h 40m (was: 1.5h) > StopWatch: steps feature > > > Key: LANG-1504 > URL: https://issues.apache.org/jira/browse/LANG-1504 > Project: Commons Lang > Issue Type: New Feature > Components: lang.time.* >Reporter: Topera >Priority: Major > Time Spent: 1h 40m > Remaining Estimate: 0h > > Would be great if we could use StopWatch to track times on many places of our > code. > I created a new feature called "*steps*". > > With this feature we can add label to each step and then get a report with > time between each step. > > Example: > {code:java} > final StopWatch watch = new StopWatch(); > watch.step("starting"); > exampleGoSleep(); > watch.step("sleeping"); > exampleGoWalk(); > watch.step("walking "); > exampleGoDance(); > watch.step("dancing "); > System.out.println(watch.getStepsReport()); > {code} > The output would be: > {noformat} > [starting] 0ms > [sleeping] 235ms > [walking ] 20ms > [dancing ] 458ms > {noformat} -- This message was sent by Atlassian Jira (v8.3.4#803005)
[jira] [Work logged] (LANG-1518) MethodUtils.getAnnotation() with searchSupers = true does not work if super is generic
[ https://issues.apache.org/jira/browse/LANG-1518?focusedWorklogId=391105=com.atlassian.jira.plugin.system.issuetabpanels:worklog-tabpanel#worklog-391105 ] ASF GitHub Bot logged work on LANG-1518: Author: ASF GitHub Bot Created on: 22/Feb/20 13:28 Start Date: 22/Feb/20 13:28 Worklog Time Spent: 10m Work Description: garydgregory commented on pull request #494: [LANG-1518] MethodUtils.getAnnotation() with searchSupers = true does not work if super is generic URL: https://github.com/apache/commons-lang/pull/494 This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org Issue Time Tracking --- Worklog Id: (was: 391105) Time Spent: 2h 10m (was: 2h) > MethodUtils.getAnnotation() with searchSupers = true does not work if super > is generic > -- > > Key: LANG-1518 > URL: https://issues.apache.org/jira/browse/LANG-1518 > Project: Commons Lang > Issue Type: Bug > Components: lang.reflect.* >Affects Versions: 3.9 >Reporter: Michele Preti >Priority: Major > Fix For: 3.10 > > Time Spent: 2h 10m > Remaining Estimate: 0h > > > {code:java} > public static class Foo { > @Nonnull > protected void test(T i) { > System.out.println("foo" + i); > } > } > public static class Bar extends Foo { > @Override > protected void test(Integer i) { > System.out.println("bar" + i); > } > } > public static void main(String[] args) throws NoSuchMethodException, > SecurityException { > Method testMethod = Bar.class.getDeclaredMethod("test", Integer.class); > > System.out.println(MethodUtils.getAnnotation(testMethod, Nonnull.class, > true, true)); //==null > } > {code} > > the method MethodUtils.getAnnotation() should be modified as souch: > (using > *MethodUtils.getMatchingMethod*/*MethodUtils.getMatchingAccessibleMethod* > instead of *getDeclaredMethod*/*getMethod*) > {code:java} > public static A getAnnotation(final Method method, > final Class annotationCls, > final boolean searchSupers, final boolean ignoreAccess) { > Validate.isTrue(method != null, "The method must not be null"); > Validate.isTrue(annotationCls != null, "The annotation class must not > be null"); > if (!ignoreAccess && !MemberUtils.isAccessible(method)) { > return null; > } > A annotation = method.getAnnotation(annotationCls); > if (annotation == null && searchSupers) { > final Class mcls = method.getDeclaringClass(); > final List> classes = > getAllSuperclassesAndInterfaces(mcls); > for (final Class acls : classes) { > Method equivalentMethod = (ignoreAccess > ? MethodUtils.getMatchingMethod(acls, > method.getName(), method.getParameterTypes()) > : > MethodUtils.getMatchingAccessibleMethod(acls, method.getName(), > method.getParameterTypes())); > if (equivalentMethod == null) { > continue; > } > annotation = > equivalentMethod.getAnnotation(annotationCls); > if (annotation != null) { > break; > } > } > } > return annotation; > } > {code} > > -- This message was sent by Atlassian Jira (v8.3.4#803005)
[jira] [Resolved] (LANG-1518) MethodUtils.getAnnotation() with searchSupers = true does not work if super is generic
[ https://issues.apache.org/jira/browse/LANG-1518?page=com.atlassian.jira.plugin.system.issuetabpanels:all-tabpanel ] Gary D. Gregory resolved LANG-1518. --- Fix Version/s: 3.10 Resolution: Fixed In git master. > MethodUtils.getAnnotation() with searchSupers = true does not work if super > is generic > -- > > Key: LANG-1518 > URL: https://issues.apache.org/jira/browse/LANG-1518 > Project: Commons Lang > Issue Type: Bug > Components: lang.reflect.* >Affects Versions: 3.9 >Reporter: Michele Preti >Priority: Major > Fix For: 3.10 > > Time Spent: 2h 10m > Remaining Estimate: 0h > > > {code:java} > public static class Foo { > @Nonnull > protected void test(T i) { > System.out.println("foo" + i); > } > } > public static class Bar extends Foo { > @Override > protected void test(Integer i) { > System.out.println("bar" + i); > } > } > public static void main(String[] args) throws NoSuchMethodException, > SecurityException { > Method testMethod = Bar.class.getDeclaredMethod("test", Integer.class); > > System.out.println(MethodUtils.getAnnotation(testMethod, Nonnull.class, > true, true)); //==null > } > {code} > > the method MethodUtils.getAnnotation() should be modified as souch: > (using > *MethodUtils.getMatchingMethod*/*MethodUtils.getMatchingAccessibleMethod* > instead of *getDeclaredMethod*/*getMethod*) > {code:java} > public static A getAnnotation(final Method method, > final Class annotationCls, > final boolean searchSupers, final boolean ignoreAccess) { > Validate.isTrue(method != null, "The method must not be null"); > Validate.isTrue(annotationCls != null, "The annotation class must not > be null"); > if (!ignoreAccess && !MemberUtils.isAccessible(method)) { > return null; > } > A annotation = method.getAnnotation(annotationCls); > if (annotation == null && searchSupers) { > final Class mcls = method.getDeclaringClass(); > final List> classes = > getAllSuperclassesAndInterfaces(mcls); > for (final Class acls : classes) { > Method equivalentMethod = (ignoreAccess > ? MethodUtils.getMatchingMethod(acls, > method.getName(), method.getParameterTypes()) > : > MethodUtils.getMatchingAccessibleMethod(acls, method.getName(), > method.getParameterTypes())); > if (equivalentMethod == null) { > continue; > } > annotation = > equivalentMethod.getAnnotation(annotationCls); > if (annotation != null) { > break; > } > } > } > return annotation; > } > {code} > > -- This message was sent by Atlassian Jira (v8.3.4#803005)
[GitHub] [commons-lang] garydgregory merged pull request #494: [LANG-1518] MethodUtils.getAnnotation() with searchSupers = true does not work if super is generic
garydgregory merged pull request #494: [LANG-1518] MethodUtils.getAnnotation() with searchSupers = true does not work if super is generic URL: https://github.com/apache/commons-lang/pull/494 This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org With regards, Apache Git Services
[jira] [Updated] (LANG-1518) MethodUtils.getAnnotation() with searchSupers = true does not work if super is generic
[ https://issues.apache.org/jira/browse/LANG-1518?page=com.atlassian.jira.plugin.system.issuetabpanels:all-tabpanel ] Gary D. Gregory updated LANG-1518: -- Summary: MethodUtils.getAnnotation() with searchSupers = true does not work if super is generic (was: MethodUtils.getAnnotation() with searchSupers =true does not work if super is generic) > MethodUtils.getAnnotation() with searchSupers = true does not work if super > is generic > -- > > Key: LANG-1518 > URL: https://issues.apache.org/jira/browse/LANG-1518 > Project: Commons Lang > Issue Type: Bug > Components: lang.reflect.* >Affects Versions: 3.9 >Reporter: Michele Preti >Priority: Major > Time Spent: 2h > Remaining Estimate: 0h > > > {code:java} > public static class Foo { > @Nonnull > protected void test(T i) { > System.out.println("foo" + i); > } > } > public static class Bar extends Foo { > @Override > protected void test(Integer i) { > System.out.println("bar" + i); > } > } > public static void main(String[] args) throws NoSuchMethodException, > SecurityException { > Method testMethod = Bar.class.getDeclaredMethod("test", Integer.class); > > System.out.println(MethodUtils.getAnnotation(testMethod, Nonnull.class, > true, true)); //==null > } > {code} > > the method MethodUtils.getAnnotation() should be modified as souch: > (using > *MethodUtils.getMatchingMethod*/*MethodUtils.getMatchingAccessibleMethod* > instead of *getDeclaredMethod*/*getMethod*) > {code:java} > public static A getAnnotation(final Method method, > final Class annotationCls, > final boolean searchSupers, final boolean ignoreAccess) { > Validate.isTrue(method != null, "The method must not be null"); > Validate.isTrue(annotationCls != null, "The annotation class must not > be null"); > if (!ignoreAccess && !MemberUtils.isAccessible(method)) { > return null; > } > A annotation = method.getAnnotation(annotationCls); > if (annotation == null && searchSupers) { > final Class mcls = method.getDeclaringClass(); > final List> classes = > getAllSuperclassesAndInterfaces(mcls); > for (final Class acls : classes) { > Method equivalentMethod = (ignoreAccess > ? MethodUtils.getMatchingMethod(acls, > method.getName(), method.getParameterTypes()) > : > MethodUtils.getMatchingAccessibleMethod(acls, method.getName(), > method.getParameterTypes())); > if (equivalentMethod == null) { > continue; > } > annotation = > equivalentMethod.getAnnotation(annotationCls); > if (annotation != null) { > break; > } > } > } > return annotation; > } > {code} > > -- This message was sent by Atlassian Jira (v8.3.4#803005)
[GitHub] [commons-csv] garydgregory commented on issue #58: export page data from db
garydgregory commented on issue #58: export page data from db URL: https://github.com/apache/commons-csv/pull/58#issuecomment-589954505 This ticket has no description so I have no idea what the intent is here. The lack of Javadoc does not help either. This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org With regards, Apache Git Services
[GitHub] [commons-math] chentao106 commented on issue #118: Feature MiniBatchKMeans
chentao106 commented on issue #118: Feature MiniBatchKMeans URL: https://github.com/apache/commons-math/pull/118#issuecomment-589953927 Compare to KMeans 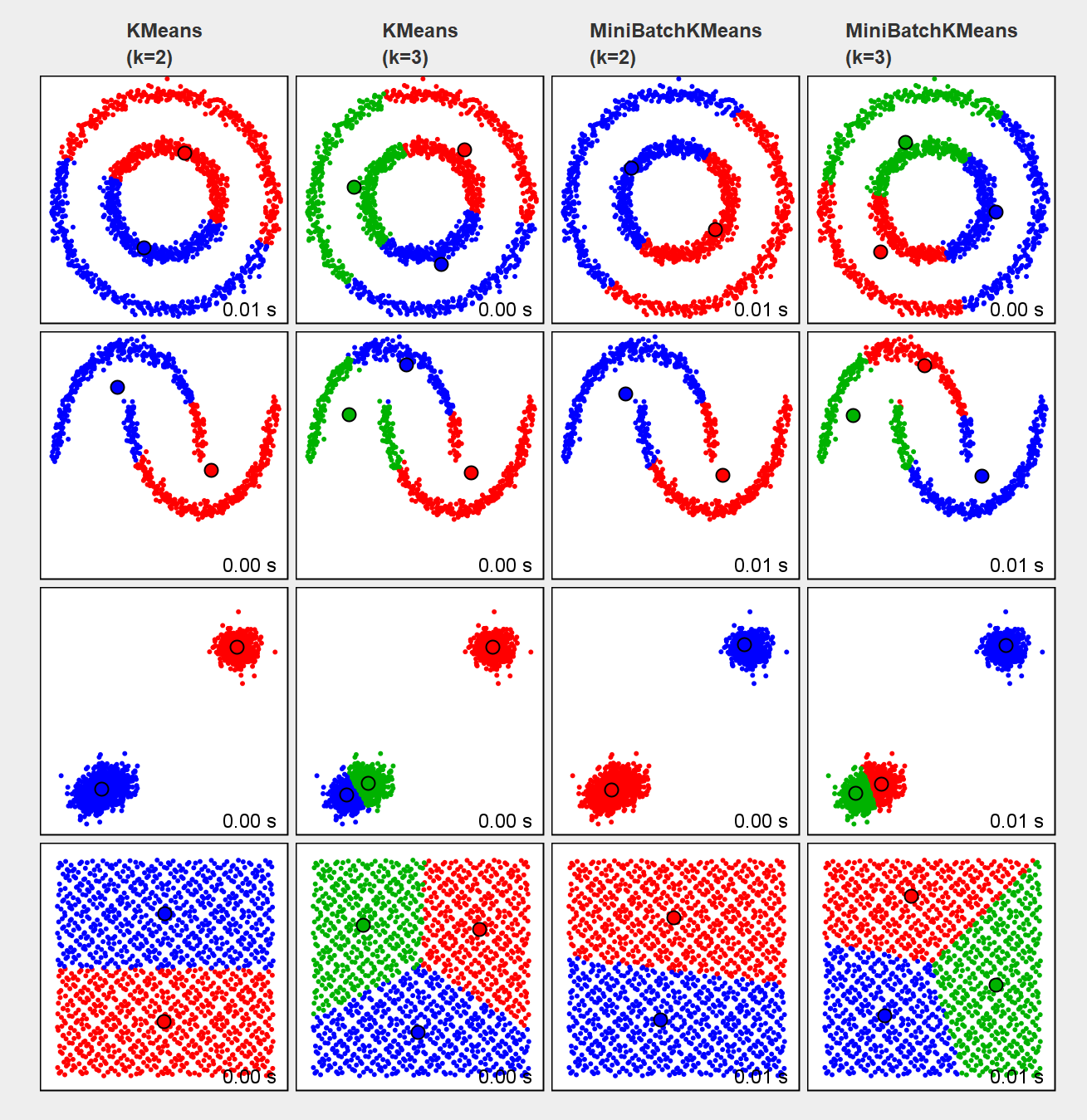 This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org With regards, Apache Git Services
[GitHub] [commons-csv] shootercheng commented on issue #58: export page data from db
shootercheng commented on issue #58: export page data from db URL: https://github.com/apache/commons-csv/pull/58#issuecomment-589939564 > [](https://coveralls.io/builds/28894087) > > Coverage decreased (-1.3%) to 91.879% when pulling **[f98dfe2](https://github.com/apache/commons-csv/commit/f98dfe2e8b8169c42e7aaa2692389dcc132f1bd9) on shootercheng:master** into **[e503c56](https://github.com/apache/commons-csv/commit/e503c568a10ae42364a94695b8505bcb4db28969) on apache:master**. 所有的方法都测试了,这个覆盖率检测有bug This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org With regards, Apache Git Services
[GitHub] [commons-csv] coveralls edited a comment on issue #58: export page data from db
coveralls edited a comment on issue #58: export page data from db URL: https://github.com/apache/commons-csv/pull/58#issuecomment-589916433 [](https://coveralls.io/builds/28894087) Coverage decreased (-1.3%) to 91.879% when pulling **f98dfe2e8b8169c42e7aaa2692389dcc132f1bd9 on shootercheng:master** into **e503c568a10ae42364a94695b8505bcb4db28969 on apache:master**. This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org With regards, Apache Git Services
[GitHub] [commons-csv] coveralls edited a comment on issue #58: export page data from db
coveralls edited a comment on issue #58: export page data from db URL: https://github.com/apache/commons-csv/pull/58#issuecomment-589916433 [](https://coveralls.io/builds/28894030) Coverage decreased (-1.3%) to 91.879% when pulling **ebe4a60a21e47125170cb17ab51f753f5b290d83 on shootercheng:master** into **e503c568a10ae42364a94695b8505bcb4db28969 on apache:master**. This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org With regards, Apache Git Services
[GitHub] [commons-csv] coveralls edited a comment on issue #58: export page data from db
coveralls edited a comment on issue #58: export page data from db URL: https://github.com/apache/commons-csv/pull/58#issuecomment-589916433 [](https://coveralls.io/builds/28893984) Coverage decreased (-1.9%) to 91.313% when pulling **70f6ad23ae3fd98b76e42639cc67072cd8b66e0d on shootercheng:master** into **e503c568a10ae42364a94695b8505bcb4db28969 on apache:master**. This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org With regards, Apache Git Services
[GitHub] [commons-csv] coveralls edited a comment on issue #58: export page data from db
coveralls edited a comment on issue #58: export page data from db URL: https://github.com/apache/commons-csv/pull/58#issuecomment-589916433 [](https://coveralls.io/builds/28893955) Coverage decreased (-1.9%) to 91.313% when pulling **d4b83571b0b7e8d6952232874e593113c0104381 on shootercheng:master** into **e503c568a10ae42364a94695b8505bcb4db28969 on apache:master**. This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org With regards, Apache Git Services
[GitHub] [commons-csv] coveralls edited a comment on issue #58: export page data from db
coveralls edited a comment on issue #58: export page data from db URL: https://github.com/apache/commons-csv/pull/58#issuecomment-589916433 [](https://coveralls.io/builds/28893848) Coverage decreased (-2.9%) to 90.368% when pulling **ed6a7fea2dff59866dfefbbbcd9977d61be3428c on shootercheng:master** into **e503c568a10ae42364a94695b8505bcb4db28969 on apache:master**. This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org With regards, Apache Git Services
[GitHub] [commons-csv] coveralls edited a comment on issue #58: export page data from db
coveralls edited a comment on issue #58: export page data from db URL: https://github.com/apache/commons-csv/pull/58#issuecomment-589916433 [](https://coveralls.io/builds/28893736) Coverage decreased (-4.8%) to 88.385% when pulling **9e1760fd820e2952943de1ae1f53ceb1d738ce76 on shootercheng:master** into **e503c568a10ae42364a94695b8505bcb4db28969 on apache:master**. This is an automated message from the Apache Git Service. To respond to the message, please log on to GitHub and use the URL above to go to the specific comment. For queries about this service, please contact Infrastructure at: us...@infra.apache.org With regards, Apache Git Services